Python: Get the third side of right angled triangle from two given sides
Right Triangle Third Side
Write a Python program to get the third side of a right-angled triangle from two given sides.
Note: Use bitwise operations to add two numbers.
Visual Presentation:
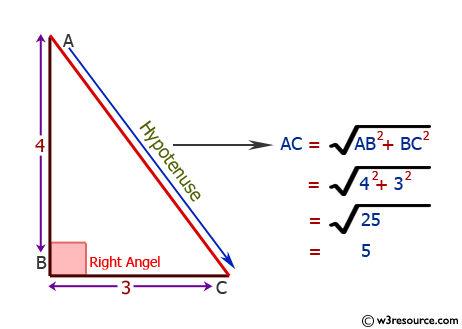
Sample Solution:
Python Code:
# Define a function 'pythagoras' that calculates the missing side of a right-angled triangle.
def pythagoras(opposite_side, adjacent_side, hypotenuse):
# Check if the opposite side is marked as unknown.
if opposite_side == str("x"):
return ("Opposite = " + str(((hypotenuse**2) - (adjacent_side**2))**0.5))
# Check if the adjacent side is marked as unknown.
elif adjacent_side == str("x"):
return ("Adjacent = " + str(((hypotenuse**2) - (opposite_side**2))**0.5))
# Check if the hypotenuse is marked as unknown.
elif hypotenuse == str("x"):
return ("Hypotenuse = " + str(((opposite_side**2) + (adjacent_side**2))**0.5))
else:
return "You know the answer!" # Return this message if all sides are known.
# Test the function with different inputs and print the results.
print(pythagoras(3, 4, 'x'))
print(pythagoras(3, 'x', 5))
print(pythagoras('x', 4, 5))
print(pythagoras(3, 4, 5))
Sample Output:
Hypotenuse = 5.0 Adjacent = 4.0 Opposite = 3.0 You know the answer!
Explanation:
The above Python code defines a function called "pythagoras()" that calculates the missing side of a right-angled triangle using the Pythagorean theorem. Here's a brief explanation:
- The function takes three arguments: 'opposite_side', 'adjacent_side', and 'hypotenuse'. One of these sides is marked as 'x', indicating it is unknown and needs to be calculated.
- The function uses conditional statements to check which side is marked as 'x' and calculates its value based on the Pythagorean theorem.
- If the opposite side is marked as 'x', it calculates and returns the value of the opposite side using the theorem.
- If the adjacent side is marked as 'x', it calculates and returns the value of the adjacent side.
- If the hypotenuse is marked as 'x', it calculates and returns the value of the hypotenuse.
- If none of the sides is marked as 'x', it returns the message "You know the answer!" since all sides are known.
- The code then tests the "pythagoras()" function with different inputs, representing various scenarios of known and unknown sides, and prints the results.
Flowchart:
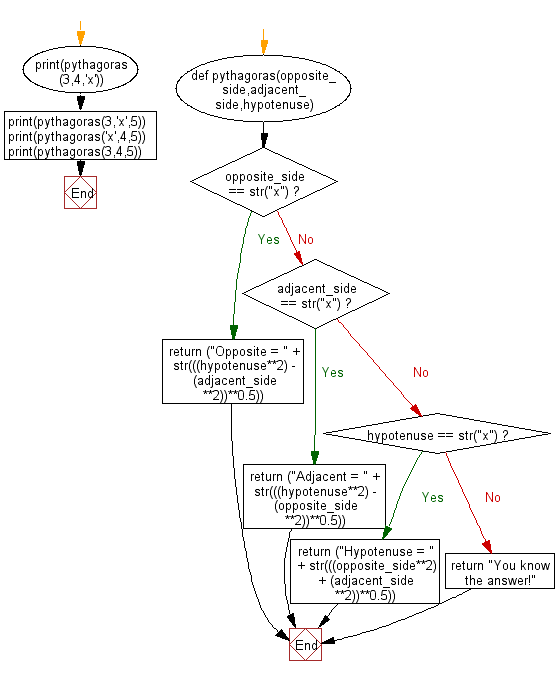
For more Practice: Solve these Related Problems:
- Write a Python program to verify if three provided numbers can form a right-angled triangle.
- Write a Python program to compute the area of a right-angled triangle given the lengths of two sides.
- Write a Python program to determine the missing leg of a right triangle when given the hypotenuse and one leg.
- Write a Python program to calculate all interior angles of a right triangle given two side lengths.
Go to:
Previous: Write a Python program to check the priority of the four operators (+, -, *, /).
Next: Write a Python program to get all strobogrammatic numbers that are of length n.
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.