Python Challenges: Find majority element in a list
Write a Python program to find majority element in a list.
Note: The majority element is the element that appears more than n/2 times where n is the number of elements in the list.
Explanation:
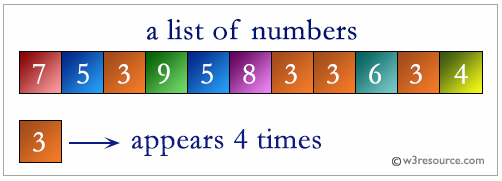
Sample Solution:
Python Code:
def majority_element(num_list):
idx, ctr = 0, 1
for i in range(1, len(num_list)):
if num_list[idx] == num_list[i]:
ctr += 1
else:
ctr -= 1
if ctr == 0:
idx = i
ctr = 1
return num_list[idx]
print(majority_element([1, 2, 3, 4, 5, 5, 5, 5, 5, 5, 6]))
print(majority_element([1,2,3,4,3,3,2,4,5,6,1,2,3,4,6,1,2,3,4,6,6]))
Sample Output:
5 6
Flowchart:
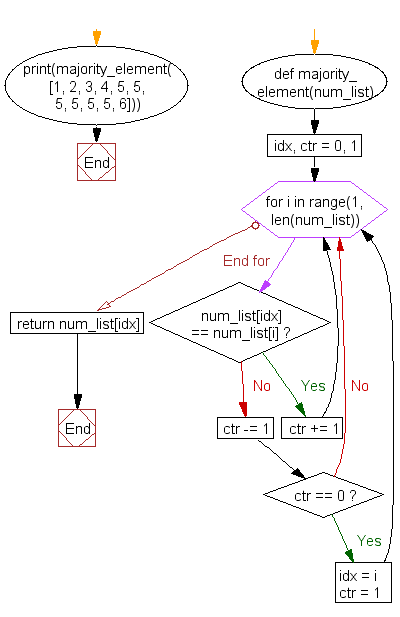
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to the push the first number to the end of a list.
Next: Write a Python program to find the length of the last word.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.