Python Challenges: Compute the sum of the even-valued terms in the Fibonacci sequence
Python Challenges - 1: Exercise-34 with Solution
Write a Python program to compute the sum of the even-valued terms in the Fibonacci sequence whose values do not exceed one million.
Note: Fibonacci series is generated by adding the previous two terms. By starting with 1 and 2, the first 10 terms will be: 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, ...
Sample Solution:
Python Code:
cache = {}
def fiba(n):
cache[n] = cache.get(n, 0) or (n <= 1 and 1 or fiba(n-1) + fiba(n-2))
return cache[n]
n = 0
x = 0
while fiba(x) <= 1000000:
if not fiba(x) % 2: n = n + fiba(x)
x=x+1
print(n)
Sample Output:
1089154
Flowchart:
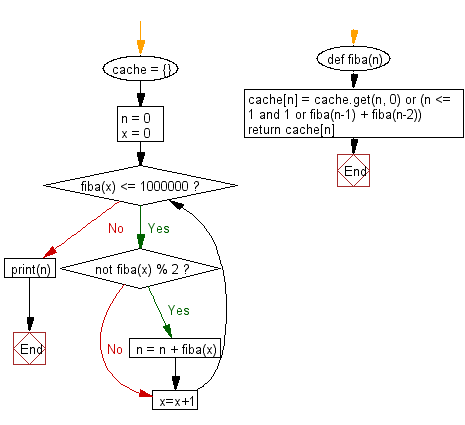
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to compute the sum of all the multiples of 3 or 5 below 500.
Next: Write a Python program to find the largest prime factor of a given number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/challenges/1/python-challenges-1-exercise-34.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics