Python Challenges: Find the starting number, under ten thousand will create the longest chain
Python Challenges - 1: Exercise-42 with Solution
Write a Python program to find the starting number, under ten thousand will create the longest chain.
From Wikipedia - The Collatz conjecture is a conjecture in mathematics that concerns a sequence defined as follows: start with any positive integer n. Then each term is obtained from the previous term as follows: if the previous term is even, the next term is one half the previous term. If the previous term is odd, the next term is 3 times the previous term plus 1. The conjecture is that no matter what value of n, the sequence will always reach 1.
Sample Solution:
Python Code:
def create_sequence_num(n):
terms = 1
while n > 1:
if n % 2 == 0:
n = n / 2
else:
n = 3 * n + 1
terms += 1
return terms
def Collatz_starting_num():
temp = 0
i = 1
while i < 10000:
s = create_sequence_num(i)
if s > temp:
temp = s
value = i
i += 1
return value
print(Collatz_starting_num())
Sample Output:
6171
Flowchart:
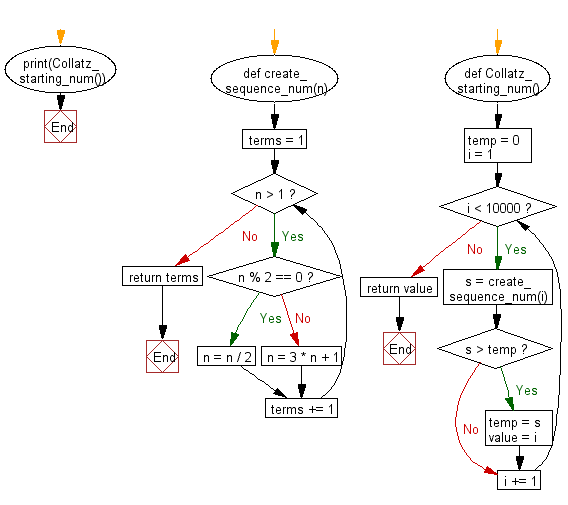
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to find the first triangle number to have over n(given) divisors.
Next: Write a Python program to compute s the sum of the digits of the number 220.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/challenges/1/python-challenges-1-exercise-42.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics