Python Challenges: Compute the sum of all the amicable numbers under a given number
Write a Python program to compute the sum of all the amicable numbers under a given number.
From Wikipedia - Amicable numbers are two different numbers so related that the sum of the proper divisors of each is equal to the other number. (A proper divisor of a number is a positive factor of that number other than the number itself. For example, the proper divisors of 6 are 1, 2, and 3.)
The smallest pair of amicable numbers is (220, 284). They are amicable because the proper divisors of 220 are 1, 2, 4, 5, 10, 11, 20, 22, 44, 55 and 110, of which the sum is 284; and the proper divisors of 284 are 1, 2, 4, 71 and 142, of which the sum is 220.
Sample Solution:
Python Code:
def amicable_sum(num):
divisor_sum = [0] * num
for i in range(1, len(divisor_sum)):
for j in range(i * 2, len(divisor_sum), i):
divisor_sum[j] += i
# Find all amicable pairs
result = 0
for i in range(1, len(divisor_sum)):
j = divisor_sum[i]
if j != i and j < len(divisor_sum) and divisor_sum[j] == i:
result += i
return str(result)
print(amicable_sum(500))
print(amicable_sum(10000))
Sample Output:
504 31626
Flowchart:
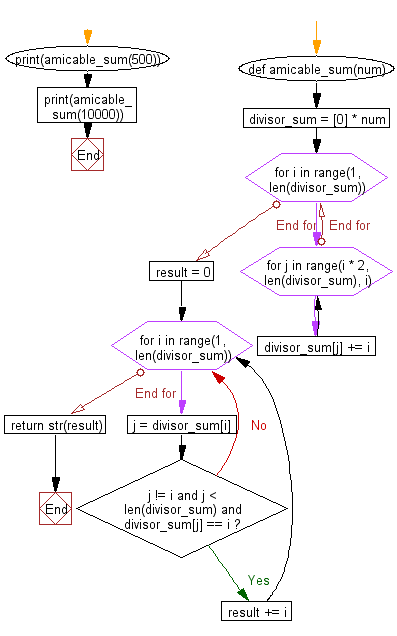
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to find the sum of the digits of a given number.
Next: Write a Python program to find the sum of all the positive integers which cannot be written as the sum of two abundant numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.