Python Challenges: Get number of distinct terms generated by ab
Consider all integer combinations of ab for 2 ≤ a ≤ 5 and 2 ≤ b ≤ 5:
22=4, 23=8, 24=16, 25=32
32=9, 33=27, 34=81, 35=243
42=16, 43=64, 44=256, 45=1024
52=25, 53=125, 54=625, 55=3125
If they are then placed in numerical order, with any repeats removed, we get the following sequence of 15 distinct terms:
4, 8, 9, 16, 25, 27, 32, 64, 81, 125, 243, 256, 625, 1024, 3125
Write a Python program to get number of distinct terms generated by ab for 2 ≤ a ≤ 21 and 2 ≤ b ≤ 21. Also print all the distinct numbers.
Sample Solution:
Python Code:
def compute_distinct_num():
nums = set(a**b for a in range(2, 21) for b in range(2, 21))
return str(len(nums)), nums
x,y = compute_distinct_num()
print("Number of distinct elements:", x)
print("\nDistinct elements are:")
print(y)
Sample Output:
Number of distinct elements: 324 Distinct elements are: {512, 1024, 2048, 4096, 4, 8192, 32768, 65536, 8, 131072, 262144, 524288, 9, 1048576, 274877906944, 1099511627776, 16, 26623333280885243904, 38416, 30491346729331195904, 332525673007965087890625, 10000000000000000000, 16384, 104976, 104127350297911241532841, 25, 31381059609, 27, 5480386857784802185939, 262144000000000000000000, 16777216, 32, 67108864, 268435456, 1073741824, 4294967296, 17179869184, 68719476736, 36, 13841287201, 12157665459056928801, 1771561, 100000000000000000000, 18446744073709551616, 75557863725914323419136, 1208925819614629174706176, 95367431640625, 10077696, 49, 121439531096594251776, 3125, 2213314919066161, 4747561509943, 3570467226624, 13107200000000000000000, 34522712143931, 793714773254144, 3656158440062976, 64, 46656, 1000000, 665416609183179841, 7529536, 4722366482869645213696, 2821109907456, 10000000000000000, 429981696, 2177953337809371136, 83521, 505447028499293771, 2476099, 25600000000, 20822964865671168, 1461920290375446110677, 759375, 81, 244140625, 678223072849, 1000000000, 1419857, 4064231406647572522401601, 12748236216396078174437376, 371293, 2097152, 7776, 248832, 576650390625, 1477891880035400390625, 100, 612220032, 14348907, 15407021574586368, 625, 20661046784, 827240261886336764177, 121, 893871739, 125, 3833759992447475122176, 128, 2176782336, 1853020188851841, 10000000, 1000000000000, 2985984, 100000000000000000, 232630513987207, 56693912375296, 16677181699666569, 379749833583241, 2187, 319479999370622926848, 295147905179352825856, 160000, 38443359375, 144, 3138428376721, 118587876497, 198359290368, 204800000000000, 2197, 100000000000000, 1977326743, 8589934592, 549755813888, 4398046511104, 4194304, 35184372088832, 281474976710656, 2251799813685248, 18014398509481984, 144115188075855872, 1152921504606846976, 100000, 11390625, 39346408075296537575424, 59049, 169, 64000000, 35831808, 2744, 205891132094649, 4826809, 426878854210636742656, 512000000000, 288441413567621167681, 1728, 362797056, 5764801, 45949729863572161, 196, 762939453125, 582622237229761, 5832, 6859, 1946195068359375, 19004963774880799438801, 150094635296999121, 9904578032905937, 1280000000, 216, 729, 25937424601, 48828125, 537824, 79792266297612001, 282429536481, 19683, 225, 15181127029874798299, 155568095557812224, 96889010407, 5976303958948914397184, 282475249, 243, 823543, 9765625, 47045881, 256, 1679616, 13060694016, 100000000, 10000000000000, 20736, 101559956668416, 1000000000000000000, 5159780352, 15625, 1475789056, 6568408355712890625, 11019960576, 2218611106740436992, 17592186044416, 4503599627370496, 1296, 10000, 72057594037927936, 130321, 672749994932560009201, 11112006825558016, 48661191875666868481, 374813367582081024, 112455406951957393129, 799006685782884121, 161051, 134217728, 1978419655660313589123979, 655360000000000000000, 1889568, 60466176, 10000000000, 289, 34012224, 16983563041, 815730721, 2357947691, 116490258898219, 78125, 3375, 22876792454961, 14641, 1331, 4913, 1792160394037, 239072435685151324847153, 37589973457545958193355601, 98526125335693359375, 5559917313492231481, 19073486328125, 61917364224, 8000, 43046721, 100000000000, 129746337890625, 324, 10240000000000, 40353607, 387420489, 1156831381426176, 8650415919381337933, 285311670611, 343, 6746640616477458432, 1350851717672992089, 3200000, 106993205379072, 2401, 214358881, 24137569, 32768000000000000000, 1953125, 470184984576, 62748517, 361, 6975757441, 170859375, 410338673, 322687697779, 29192926025390625, 64268410079232, 4782969, 279936, 33232930569601, 105413504, 2562890625, 16926659444736, 6131066257801, 30517578125, 61159090448414546291, 184884258895036416, 400, 3486784401, 117649, 28561, 1220703125, 78364164096, 289254654976, 1283918464548864, 1638400000000000000, 743008370688, 6561, 2015993900449, 42052983462257059, 1000000000000000, 16807, 8916100448256, 10604499373, 4096000000000000, 8649755859375, 104857600000000000000000000, 1628413597910449, 23298085122481, 34271896307633, 2185911559738696531968, 51185893014090757, 83668255425284801560576, 22168378200531005859375, 152587890625, 4049565169664, 129140163, 19487171, 50625, 5242880000000000000000000, 137858491849, 708235345355337676357632, 609359740010496, 1594323, 11398895185373143, 3814697265625, 3937376385699289, 1162261467, 81920000000000000, 390625, 168377826559400929, 4177248169415651, 1000, 6103515625, 2541865828329, 437893890380859375, 531441, 2862423051509815793, 14063084452067724991009, 177147, 302875106592253}
Flowchart:
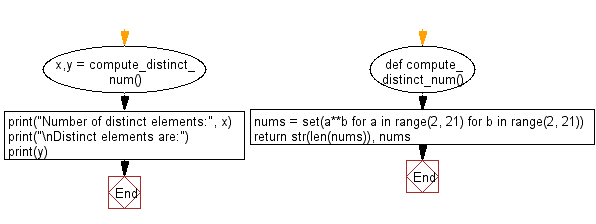
#Ref. https://bit.ly/2vaWX1y
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to compute the sum of the numbers on the diagonals in a 1001 by 1001 spiral formed.
Next: Write a Python program to find the sum of all the numbers that can be written as the sum of fifth powers of their digits.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.