Python: Create an instance of a specified class and display the namespace of the said instance
Python Class ( Basic ): Exercise-3 with Solution
Write a Python program to create an instance of a specified class and display the namespace of the said instance.
Sample Solution:
Python Code:
class Student:
def __init__(self, student_id, student_name, class_name):
self.student_id = student_id
self.student_name = student_name
self.class_name = class_name
student = Student('V12', 'Frank Gibson', 'V')
print(student.__dict__)
Sample Output:
{'student_id': 'V12', 'student_name': 'Frank Gibson', 'class_name': 'V'}
Flowchart:
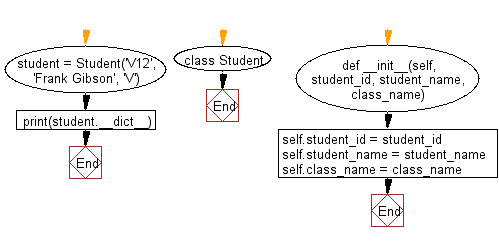
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to create a class and display the namespace of the said class.
Next: Write a python program which import the abs() function using the builtins module, display the documentation of abs() function and find the absolute value of -155.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/class-exercises/python-class-basic-1-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics