Python Class - Inventory Management System
4. Inventory Class with Item Management and Reporting
Write a Python class Inventory with attributes like item_id, item_name, stock_count, and price, and methods like add_item, update_item, and check_item_details.
Use a dictionary to store the item details, where the key is the item_id and the value is a dictionary containing the item_name, stock_count, and price
Sample Solution:
Python Code:
class Inventory:
def __init__(self):
self.inventory = {}
def add_item(self, item_id, item_name, stock_count, price):
self.inventory[item_id] = {"item_name": item_name, "stock_count": stock_count, "price": price}
def update_item(self, item_id, stock_count, price):
if item_id in self.inventory:
self.inventory[item_id]["stock_count"] = stock_count
self.inventory[item_id]["price"] = price
else:
print("Item not found in inventory.")
def check_item_details(self, item_id):
if item_id in self.inventory:
item = self.inventory[item_id]
return f"Product Name: {item['item_name']}, Stock Count: {item['stock_count']}, Price: {item['price']}"
else:
return "Item not found in inventory."
inventory = Inventory()
inventory.add_item("I001", "Laptop", 100, 500.00)
inventory.add_item("I002", "Mobile", 110, 450.00)
inventory.add_item("I003", "Desktop", 120, 500.00)
inventory.add_item("I004", "Tablet", 90, 550.00)
print("Item Details:")
print(inventory.check_item_details("I001"))
print(inventory.check_item_details("I002"))
print(inventory.check_item_details("I003"))
print(inventory.check_item_details("I004"))
print("\nUpdate the price of item code - 'I001':")
inventory.update_item("I001", 100, 505.00)
print(inventory.check_item_details("I001"))
print("\nUpdate the stock of item code - 'I003':")
inventory.update_item("I003", 115, 500.00)
print(inventory.check_item_details("I003"))
Sample Output:
Item Details: Product Name: Laptop, Stock Count: 100, Price: 500.0 Product Name: Mobile, Stock Count: 110, Price: 450.0 Product Name: Desktop, Stock Count: 120, Price: 500.0 Product Name: Tablet, Stock Count: 90, Price: 550.0 Update the price of item code - 'I001': Product Name: Laptop, Stock Count: 100, Price: 505.0 Update the stock of item code - 'I003': Product Name: Desktop, Stock Count: 115, Price: 500.0
Flowchart:
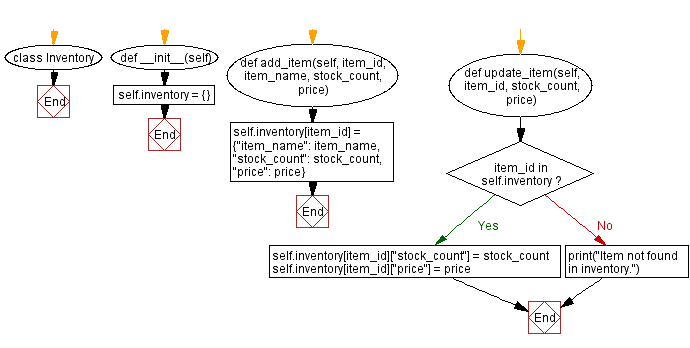
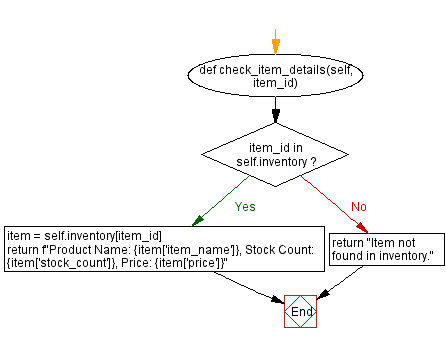
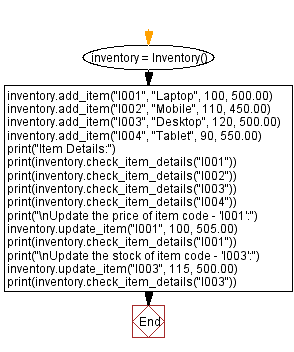
For more Practice: Solve these Related Problems:
- Write a Python class Inventory that supports adding new items, updating existing ones, and removing items; include a method to generate a report of items with stock below a certain threshold.
- Write a Python class Inventory that processes a bulk update of stock counts from a list of transactions, updating the internal dictionary accordingly.
- Write a Python class Inventory that calculates and returns the total inventory value by multiplying each item's stock_count by its price, and then summing the totals.
- Write a Python class Inventory that implements a search function to find items by a substring in the item_name using regular expressions, and prints matching items with their details.
Go to:
Previous: Bank Account Management.
Next: Python Unit Test Exercises Home.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.