Python: Find the characters which occur in more than and less than a given number in list of strings
Python Collections: Exercise-25 with Solution
Write a Python program to find the characters in a list of strings that occur more or less than a given number.
Sample Solution:
Python Code:
# Import the Counter class from the collections module
from collections import Counter
# Import the chain function from the itertools module
from itertools import chain
# Define a function 'max_aggregate' that finds characters occurring more or less than 'N' times in a list of strings
def max_aggregate(list_str, N):
# Create a generator 'temp' that converts each string into a set of unique characters
temp = (set(sub) for sub in list_str)
# Count the occurrences of characters across all sets using Counter and chain them together
counts = Counter(chain.from_iterable(temp))
# Create a list 'gt_N' containing characters occurring more than 'N' times
gt_N = [chr for chr, count in counts.items() if count > N]
# Create a list 'lt_N' containing characters occurring less than 'N' times
lt_N = [chr for chr, count in counts.items() if count < N]
# Return the characters occurring more and less than 'N' times
return gt_N, lt_N
# Create a list of strings 'list_str'
list_str = ['abcd', 'iabhef', 'dsalsdf', 'sdfsas', 'jlkdfgd']
# Print a message to indicate the display of the original list
print("Original list:")
# Print the content of 'list_str'
print(list_str)
# Define an integer 'N'
N = 3
# Call the 'max_aggregate' function with 'list_str' and 'N' and store the result in 'result'
result = max_aggregate(list_str, N)
# Print a message to indicate the display of characters occurring more than 'N' times
print("\nCharacters of the said list of strings which occur more than:", N)
# Print the characters from 'result[0]'
print(result[0])
# Print a message to indicate the display of characters occurring less than 'N' times
print("\nCharacters of the said list of strings which occur less than:", N)
# Print the characters from 'result[1]'
print(result[1])
Sample Output:
Original list: ['abcd', 'iabhef', 'dsalsdf', 'sdfsas', 'jlkdfgd'] Characters of the said list of strings which occur more than: 3 ['a', 'd', 'f'] Characters of the said list of strings which occur less than: 3 ['c', 'b', 'h', 'e', 'i', 's', 'l', 'k', 'j', 'g']
Flowchart:
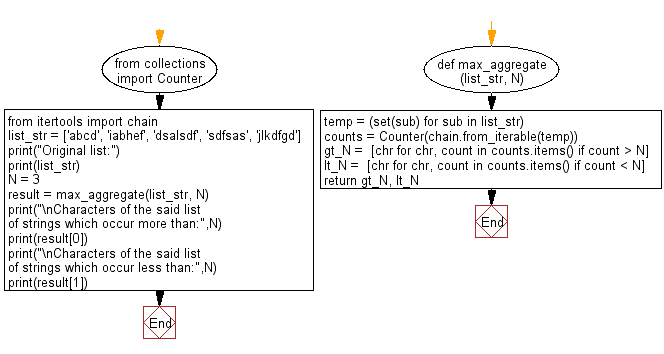
Python Code Editor:
Previous: Write a Python program to calculate the maximum aggregate from the list of tuples (pairs).
Next: Write a Python program to find the difference between two list including duplicate elements. Use collections module.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics