Python Cyber Security - Hash Password String using SHA-256 Algorithm
1. SHA-256 Hasher
Write a Python program that defines a function and takes a password string as input and returns its SHA-256 hashed representation as a hexadecimal string.
With this code, passwords can be securely stored and authenticated by hashing them and storing only their hashed representation.
Sample Solution:
Python Code:
import hashlib
def hash_password(password):
# Encode the password as bytes
password_bytes = password.encode('utf-8')
# Use SHA-256 hash function to create a hash object
hash_object = hashlib.sha256(password_bytes)
# Get the hexadecimal representation of the hash
password_hash = hash_object.hexdigest()
return password_hash
password = input("Input your password: ")
hashed_password = hash_password(password)
print(f"Your hashed password is: {hashed_password}")
Sample Output:
Input your password: A123$Loi Your hashed password is: 859b848f7f4ebf5e0c47befe74b6cb27caf5ea6a63a566f7038e24f1d29ab131
The function first encodes the password string as bytes using UTF-8 encoding and then creates a SHA-256 hash object with the hashlib.sha256 method. It then passes the encoded password bytes to this hash object by the update method. Finally, it gets the hexadecimal representation of the hash using the hexdigest method.
In the main program, the user is prompted to input their password using the input method. The hash_password function is then called with this password as the argument to generate its hashed representation. The hashed password is then printed to the console using the print method.
Python hashlib module
hashlib - Secure hashes and message digests
This module implements a common interface to many different secure hash and message digest algorithms. Included are the FIPS secure hash algorithms SHA1, SHA224, SHA256, SHA384, and SHA512 (defined in FIPS 180-2) as well as RSA’s MD5 algorithm (defined in internet RFC 1321). The terms “secure hash” and “message digest” are interchangeable. Older algorithms were called message digests. The modern term is secure hash.
Flowchart:
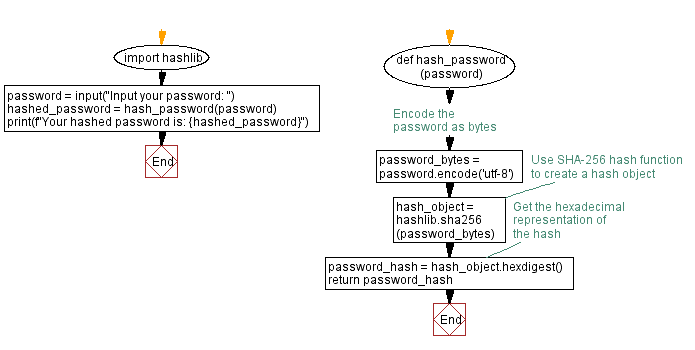
For more Practice: Solve these Related Problems:
- Write a Python function that takes a password string and returns its SHA-256 hash using the hashlib module, ensuring that the hex digest is in lowercase.
- Write a Python script to prompt the user for a password, hash it using SHA-256, and then print both the original and hashed values.
- Write a Python program to compare the SHA-256 hashes of two user-input passwords and print a message indicating whether they match.
- Write a Python function that reads a password from a file, computes its SHA-256 hash, and writes the hash to another file.
Go to:
Previous: Python Cyber Security Exercise Home.
Next: Generate random passwords of specified length.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.