Python Cyber Security - Check password strength
3. Password Criteria Checker
Write a Python program to check if a password meets the following criteria:
At least 8 characters long and
Contains at least one uppercase letter, one lowercase letter, one digit, and one special character (!, @, #, $, %, or &)
If the password meets the criteria, print a message that says "Valid Password." If it doesn't meet the criteria, print a message that says "Password does not meet requirements."
Sample Solution:
Python Code:
# Solution to Exercise 3
import re
def validate_password(password):
# Check if the password has at least 8 characters
if len(password) < 8:
return False
# Check if the password contains at least one uppercase letter
if not re.search(r'[A-Z]', password):
return False
# Check if the password contains at least one lowercase letter
if not re.search(r'[a-z]', password):
return False
# Check if the password contains at least one digit
if not re.search(r'\d', password):
return False
# Check if the password contains at least one special character
if not re.search(r'[!@#$%^&*(),.?":{}|<>]', password):
return False
# If all the conditions are met, the password is valid
return True
password = input("Input your password: ")
is_valid = validate_password(password)
if is_valid:
print("Valid Password.")
else:
print("Password does not meet requirements.")
Sample Output:
Input your password: Aji1#der Valid Password. Input your password: A123d$%h Valid Password. Input your password: A12&ki1 Password does not meet requirements. Input your password: KI342$&H Password does not meet requirements.
Flowchart:
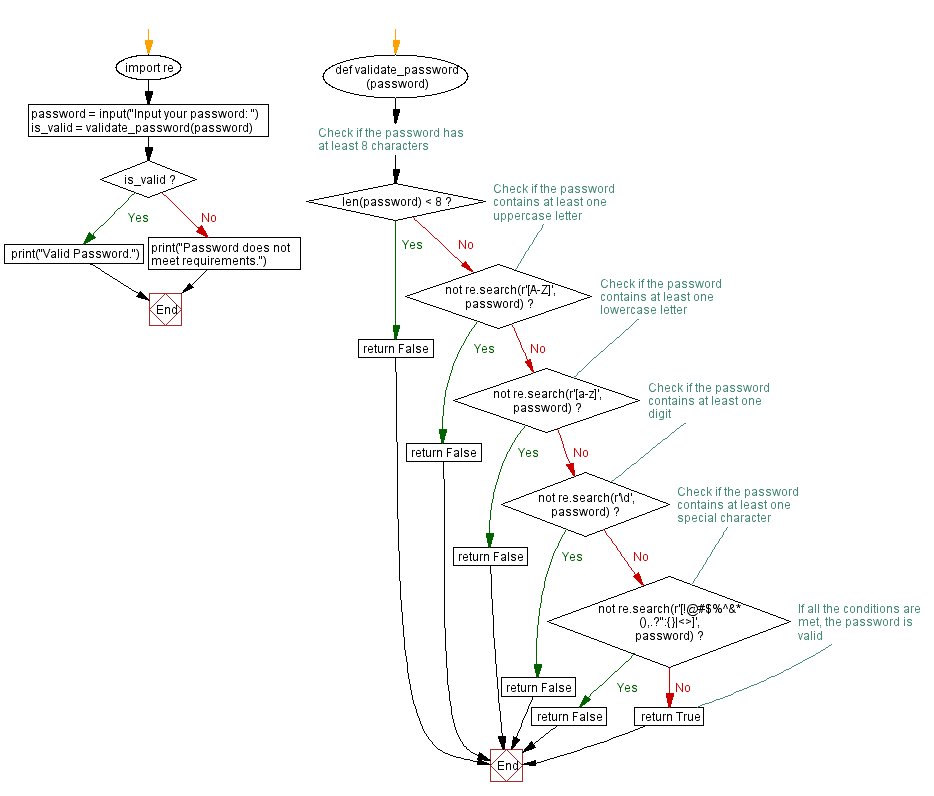
For more Practice: Solve these Related Problems:
- Write a Python program that checks if a password meets complexity requirements (minimum 8 characters, at least one uppercase, one lowercase, one digit, and one special character) and prints either "Valid Password" or "Invalid Password" with specific missing criteria.
- Write a Python function that validates a password based on given rules and returns a tuple containing a boolean flag and a list of unmet requirements.
- Write a Python script that takes a list of passwords from the user, validates each one, and then prints a report showing which passwords are valid.
- Write a Python program to simulate a password policy check that allows re-tries until a valid password is entered, then confirms success.
Go to:
Previous: Generate random passwords of specified length.
Next: Generate random passwords of specified length.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.