Python Linked List: Count the number of items of a given doubly linked list
Python Linked List: Exercise-10 with Solution
Write a Python program to count the number of items of a given doubly linked list.
Sample Solution:
Python Code:
class Node(object):
# Singly linked node
def __init__(self, data=None, next=None, prev=None):
self.data = data
self.next = next
self.prev = prev
class doubly_linked_list(object):
def __init__(self):
self.head = None
self.tail = None
self.count = 0
def append_item(self, data):
# Append an item
new_item = Node(data, None, None)
if self.head is None:
self.head = new_item
self.tail = self.head
else:
new_item.prev = self.tail
self.tail.next = new_item
self.tail = new_item
self.count += 1
items = doubly_linked_list()
items.append_item('PHP')
items.append_item('Python')
items.append_item('C#')
items.append_item('C++')
items.append_item('Java')
items.append_item('SQL')
print("Number of items of the Doubly linked list:",items.count)
Sample Output:
Number of items of the Doubly linked list: 6
Flowchart:
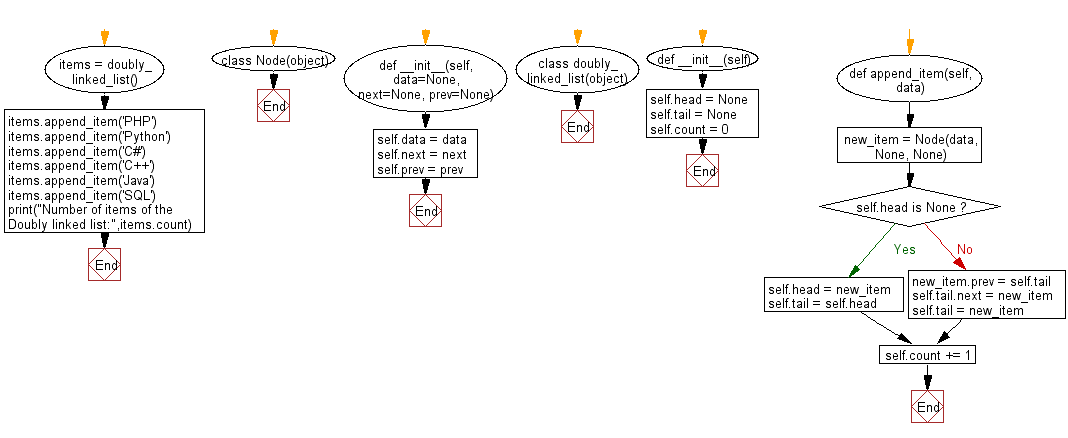
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to create a doubly linked list, append some items and iterate through the list (print forward).
Next: Write a Python program to print a given doubly linked list in reverse order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/data-structures-and-algorithms/python-linked-list-exercise-10.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics