Python Linked List: Search a specific item in a given doubly linked list and return true if the item is found otherwise return false
13. Search in Doubly Linked List
Write a Python program to search a specific item in a given doubly linked list and return true if the item is found otherwise return false.
Sample Solution:
Python Code:
class Node(object):
# Singly linked node
def __init__(self, data=None, next=None, prev=None):
self.data = data
self.next = next
self.prev = prev
class doubly_linked_list(object):
def __init__(self):
self.head = None
self.tail = None
self.count = 0
def append_item(self, data):
# Append an item
new_item = Node(data, None, None)
if self.head is None:
self.head = new_item
self.tail = self.head
else:
new_item.prev = self.tail
self.tail.next = new_item
self.tail = new_item
self.count += 1
def iter(self):
# Iterate the list
current = self.head
while current:
item_val = current.data
current = current.next
yield item_val
def print_foward(self):
for node in self.iter():
print(node)
def search_item(self, val):
for node in self.iter():
if val == node:
return True
return False
items = doubly_linked_list()
items.append_item('PHP')
items.append_item('Python')
items.append_item('C#')
items.append_item('C++')
items.append_item('Java')
items.append_item('SQL')
print("Original list:")
items.print_foward()
print("\n")
if items.search_item('SQL'):
print("True")
else:
print("False")
if items.search_item('C+'):
print("True")
else:
print("False")
Sample Output:
Original list: PHP Python C# C++ Java SQL True False
Flowchart:
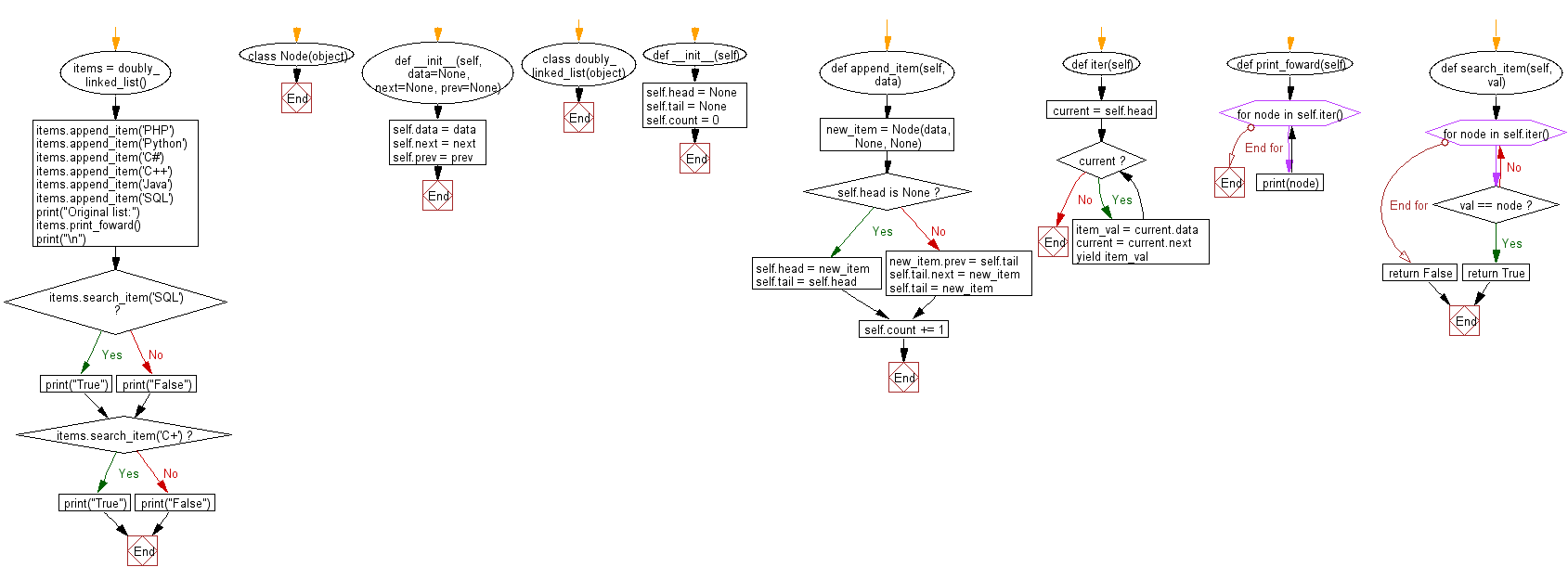
For more Practice: Solve these Related Problems:
- Write a Python program to search for a specified item in a doubly linked list and return True if found, otherwise False.
- Write a Python script to traverse a doubly linked list to locate a node with a given value and print its position.
- Write a Python program to implement a search function for a doubly linked list using both forward and backward traversal.
- Write a Python function to scan a doubly linked list for a target value and then return the node object if the value exists.
Go to:
Previous: Write a Python program to insert an item in front of a given doubly linked list.
Next: Write a Python program to delete a specific item from a given doubly linked list.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.