Python Linked List: Find the size of a singly linked list
Python Linked List: Exercise-2 with Solution
Write a Python program to find the size of a singly linked list.
Sample Solution:
Python Code:
class Node:
# Singly linked node
def __init__(self, data=None):
self.data = data
self.next = None
class singly_linked_list:
def __init__(self):
# Createe an empty list
self.tail = None
self.head = None
self.count = 0
def append_item(self, data):
#Append items on the list
node = Node(data)
if self.head:
self.head.next = node
self.head = node
else:
self.tail = node
self.head = node
self.count += 1
def iterate_item(self):
# Iterate the list.
current_item = self.tail
while current_item:
val = current_item.data
current_item = current_item.next
yield val
items = singly_linked_list()
items.append_item('PHP')
items.append_item('Python')
items.append_item('C#')
items.append_item('C++')
items.append_item('Java')
print("Original list:")
for val in items.iterate_item():
print(val)
print("\nSize of the list:",items.count)
Sample Output:
Original list: PHP Python C# C++ Java Size of the list: 5
Flowchart:
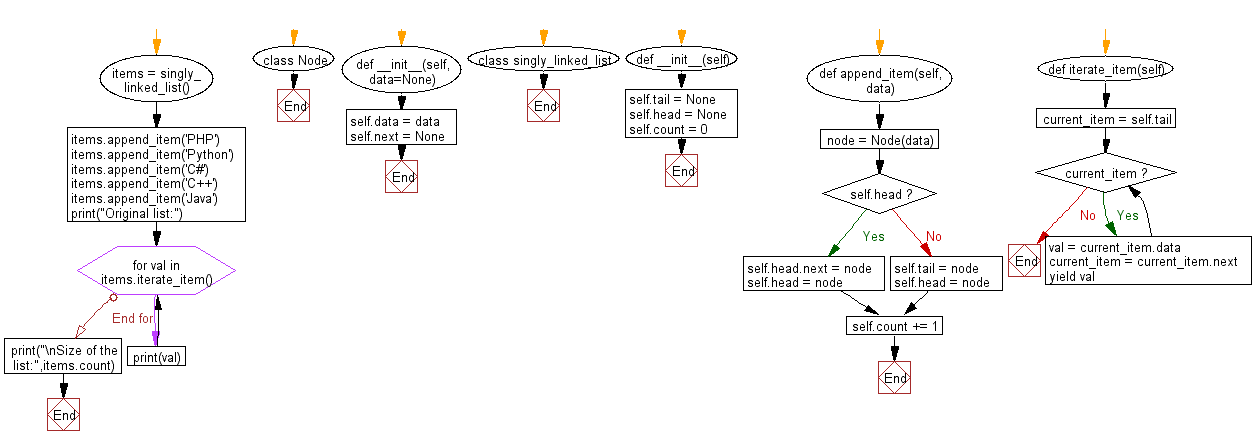
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to create a singly linked list, append some items and iterate through the list.
Next: Write a Python program to search a specific item in a singly linked list and return true if the item is found otherwise return false.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics