Python: Sort a list of elements using Radix sort
19. Radix Sort
Write a Python program to sort a list of elements using Radix sort.
According to Wikipedia "In computer science, radix sort is a non-comparative integer sorting algorithm that sorts data with integer keys by grouping keys by the individual digits which share the same significant position and value".
Sample Solution:
Python Code:
def radix_sort(nums):
RADIX = 10
placement = 1
max_digit = max(nums)
while placement < max_digit:
buckets = [list() for _ in range( RADIX )]
for i in nums:
tmp = int((i / placement) % RADIX)
buckets[tmp].append(i)
a = 0
for b in range( RADIX ):
buck = buckets[b]
for i in buck:
nums[a] = i
a += 1
placement *= RADIX
return nums
user_input = input("Input numbers separated by a comma:\n").strip()
nums = [int(item) for item in user_input.split(',')]
print(radix_sort(nums))
Sample Output:
Input numbers separated by a comma: 15, 79, 25, 68, 37 [15, 25, 37, 68, 79]
Flowchart:
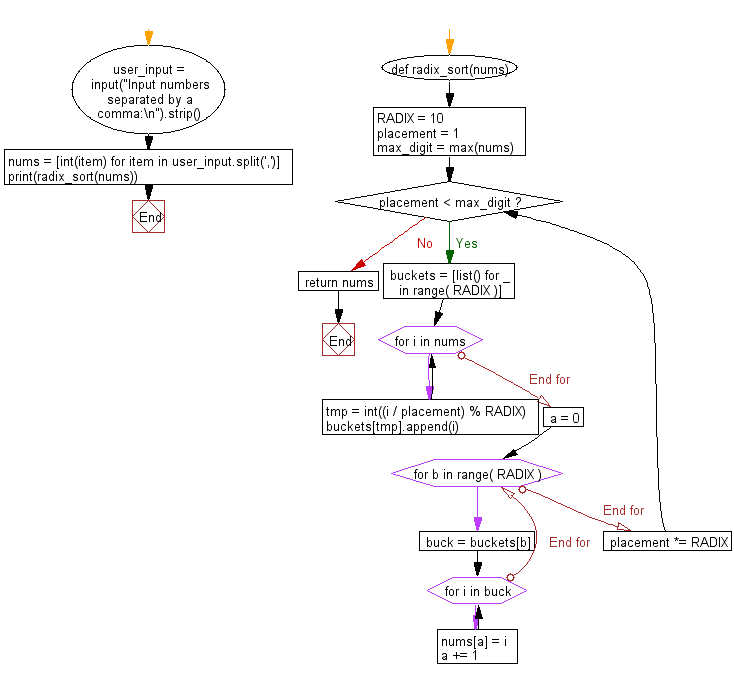
For more Practice: Solve these Related Problems:
- Write a Python program to implement radix sort for a list of non-negative integers and display the intermediate digit groupings.
- Write a Python script to sort a list of integers using radix sort and then print the sorted output along with the number of passes made.
- Write a Python program to modify radix sort to sort a list of strings representing numbers and then compare the results with numerical sorting.
- Write a Python function to implement radix sort and then validate the sorted list against Python’s built-in sorted() function.
Go to:
Previous: Write a Python program to sort a list of elements using Pancake sort.
Next: Write a Python program to sort a list of elements using Selection sort.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.