Python: Search for an ordered list
3. Ordered Binary Search
Write a Python program for binary search of an ordered list.
Sample Solution:
Python Code:
def Ordered_binary_Search(olist, item):
if len(olist) == 0:
return False
else:
midpoint = len(olist) // 2
if olist[midpoint] == item:
return True
else:
if item < olist[midpoint]:
return binarySearch(olist[:midpoint], item)
else:
return binarySearch(olist[midpoint+1:], item)
def binarySearch(alist, item):
first = 0
last = len(alist) - 1
found = False
while first <= last and not found:
midpoint = (first + last) // 2
if alist[midpoint] == item:
found = True
else:
if item < alist[midpoint]:
last = midpoint - 1
else:
first = midpoint + 1
return found
print(Ordered_binary_Search([0, 1, 3, 8, 14, 18, 19, 34, 52], 3))
print(Ordered_binary_Search([0, 1, 3, 8, 14, 18, 19, 34, 52], 17))
Sample Output:
True False
Flowchart:
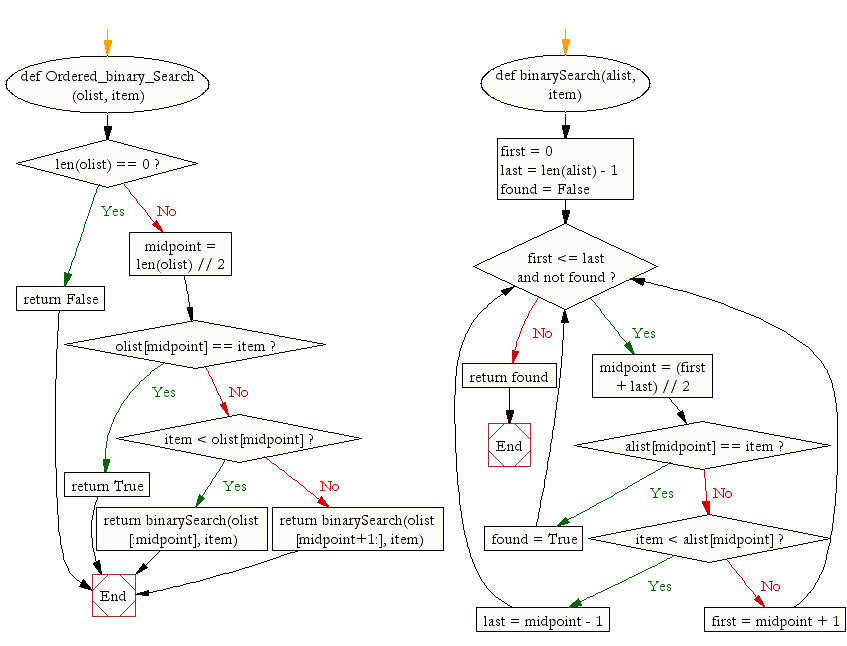
For more Practice: Solve these Related Problems:
- Write a Python program to perform binary search on an ordered list and return True if the target is found, otherwise False.
- Write a Python script to implement an iterative ordered binary search that logs each midpoint examined during the search.
- Write a Python function that uses binary search to verify the presence of a target in a sorted list and returns the number of steps required.
- Write a Python program that performs binary search on an ordered list of dates and outputs whether a given date exists in the list.
Go to:
Previous: Write a Python program for sequential search.
Next: Write a Python program to sort a list of elements using the bubble sort algorithm.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.