Python: Get last modified information of a file
Python Datetime: Exercise-38 with Solution
Write a Python program to get the last modified information of a file.
Sample Solution:
Python Code:
# Import the os and time modules
import os, time
# Define a function called last_modified_fileinfo which retrieves the last modified date and time of a file
def last_modified_fileinfo(filepath):
# Get the file status using os.stat() function
filestat = os.stat(filepath)
# Get the last modified date and time in local time
date = time.localtime((filestat.st_mtime))
# Extract year, month, and day from the date
year = date[0]
month = date[1]
day = date[2]
# Extract hour, minute, and second
hour = date[3]
minute = date[4]
second = date[5]
# Year
strYear = str(year)[0:]
# Month
if (month <=9):
strMonth = '0' + str(month)
else:
strMonth = str(month)
# Date
if (day <=9):
strDay = '0' + str(day)
else:
strDay = str(day)
# Format the date and time as a string in the format "YYYY-MM-DD HH:MM:SS"
return (strYear+"-"+strMonth+"-"+strDay+" "+str(hour)+":"+str(minute)+":"+str(second))
# Print an empty line
print()
# Print the last modified date and time of the file 'test.txt'
print(last_modified_fileinfo('test.txt'))
# Print an empty line
print()
Output:
2017-04-19 15:15:52
Explanation:
In the exercise above,
- This code imports the modules "os" and "time".
- It defines a function named "last_modified_fileinfo()" which retrieves the last modified date and time of a file specified by 'filepath'.
- Inside the "last_modified_fileinfo()" function:
- It gets the file status using the "os.stat()" function, which returns a tuple containing several pieces of information about the file, including the last modified time.
- It converts the last modified time to local time using "time.localtime()".
- It extracts the year, month, day, hour, minute, and second from the obtained date.
- It formats the date and time into a string in the format "YYYY-MM-DD HH:MM:SS".
- It prints the last modified date and time of the file 'test.txt' by calling the "last_modified_fileinfo()" function.
Flowchart:
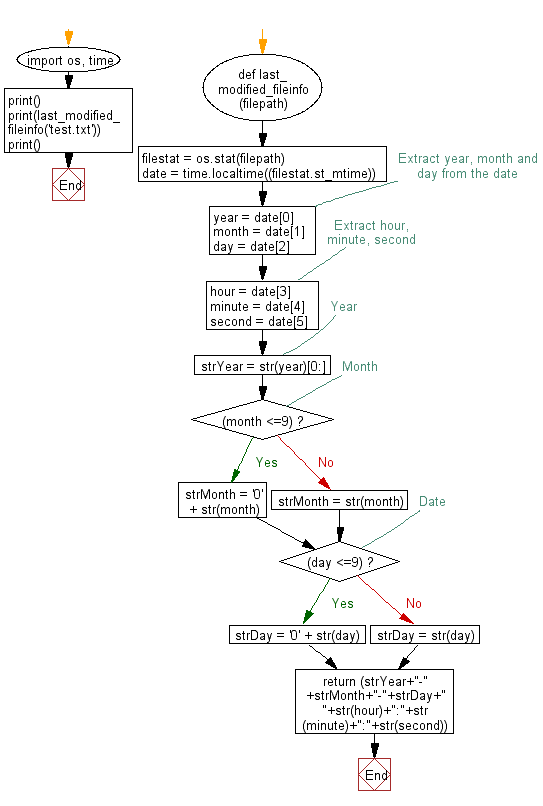
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to convert two date difference in days, hours, minutes, seconds.
Next: Write a Python program to calculate an age in year.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/date-time-exercise/python-date-time-exercise-38.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics