Python: Get a list of dates between two dates
50. Dates Between Two Dates
Write a Python program to get a list of dates between two dates.
Sample Solution:
Python Code:
# Import the timedelta and date classes from the datetime module
from datetime import timedelta, date
# Define a function named daterange which generates a range of dates between date1 and date2
def daterange(date1, date2):
# Iterate over the range of days between date1 and date2
for n in range(int((date2 - date1).days) + 1):
# Yield each date within the range
yield date1 + timedelta(n)
# Define the start date
start_dt = date(2015, 12, 20)
# Define the end date
end_dt = date(2016, 1, 11)
# Iterate over the range of dates generated by the daterange function
for dt in daterange(start_dt, end_dt):
# Print each date in the format YYYY-MM-DD
print(dt.strftime("%Y-%m-%d"))
Output:
2015-12-20 2015-12-21 2015-12-22 2015-12-23 2015-12-24 2015-12-25 2015-12-26 2015-12-27 2015-12-28 2015-12-29 2015-12-30 2015-12-31 ------- 2016-01-08 2016-01-09 2016-01-10 2016-01-11
Explanation:
In the exercise above,
- The code imports the "timedelta" and "date" classes from the datetime module.
- It defines a function named "daterange" which generates a range of dates between 'date1' and 'date2'.
- Inside the daterange function:
- It calculates the number of days between 'date1' and 'date2'.
- It iterates over the range of days using a "for" loop.
- It yields each date within the range by adding 'n' days to 'date1'.
- It defines the start date as December 20, 2015.
- It defines the end date as January 11, 2016.
- It iterates over the range of dates generated by the "daterange()" function using a "for" loop.
- Inside the loop, it prints each date in the format YYYY-MM-DD using the "strftime()" method to format the date.
Flowchart:
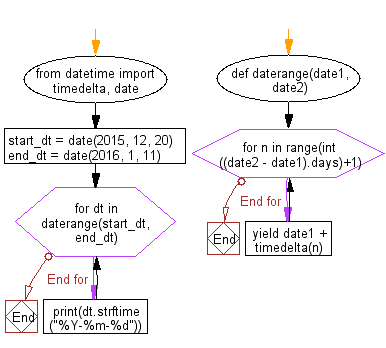
For more Practice: Solve these Related Problems:
- Write a Python program to generate a list of all dates between two given dates and then output them in a formatted list.
- Write a Python script to compute the sequence of dates between two dates and then filter out only the Mondays.
- Write a Python function to return all dates between two input dates and then count the total number of days.
- Write a Python program to generate a date range between two dates and then format each date as "Day, DD Month".
Go to:
Previous: Write a Python program to convert a string into datetime.
Next: Write a Python program to generate RFC 3339 timestamp.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.