Implementing a Python decorator for function logging
8. Implement a Decorator to Add Logging Functionality
Write a Python program that implements a decorator to add logging functionality to a function.
Logging functionality refers to the capability of recording and storing information about program execution. It allows you to capture significant events, messages, and errors that occur during code execution.
Sample Solution:
Python Code:
def add_logging(func):
def wrapper(*args, **kwargs):
# Log the function name and arguments
print(f"Calling {func.__name__} with args: {args}, kwargs: {kwargs}")
# Call the original function
result = func(*args, **kwargs)
# Log the return value
print(f"{func.__name__} returned: {result}")
# Return the result
return result
return wrapper
# Example usage
@add_logging
def add_numbers(x, y):
return x + y
# Call the decorated function
result = add_numbers(200, 300)
print("Result:", result)
Sample Output:
Calling add_numbers with args: (200, 300), kwargs: {} add_numbers returned: 500 Result: 500
Explanation:
In the above exercise,
The code defines a decorator called add_logging that adds logging functionality to a function. When a function is decorated with @add_logging, it wraps the original function and performs the following actions:
- Log the function name and arguments before calling the original function.
- Call the original function.
- Log the return value after the function execution.
- Return the result.
In the example usage, the add_numbers function is decorated with @add_logging. When the decorated function is called, the decorator logs the function name, arguments, and return value.
Flowchart:
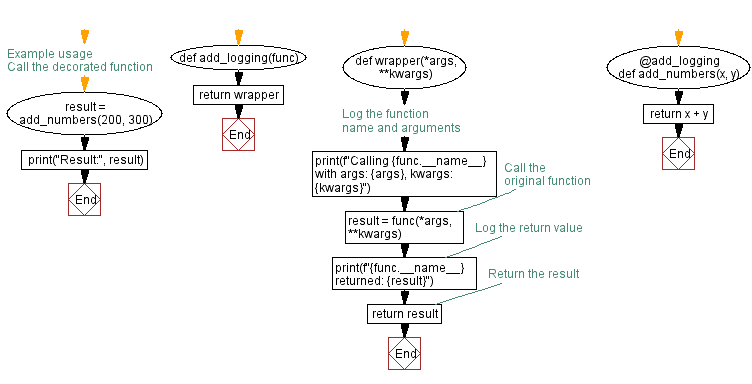
For more Practice: Solve these Related Problems:
- Write a Python decorator that logs the start and end times of a function call along with its name using the logging module.
- Write a Python decorator that writes log messages to a file every time the decorated function is invoked.
- Write a Python decorator that logs both the input arguments and the return value of a function call in a structured format.
- Write a Python decorator that integrates with the logging module to include the module and function name in each log entry.
Go to:
Previous: Implementing a Python decorator for function rate limits.
Next: Implementing a Python decorator for exception handling with a default response.
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.