Python: Filter a dictionary based on values
Python dictionary: Exercise-42 with Solution
Write a Python program to filter a dictionary based on values.
Visual Presentation:
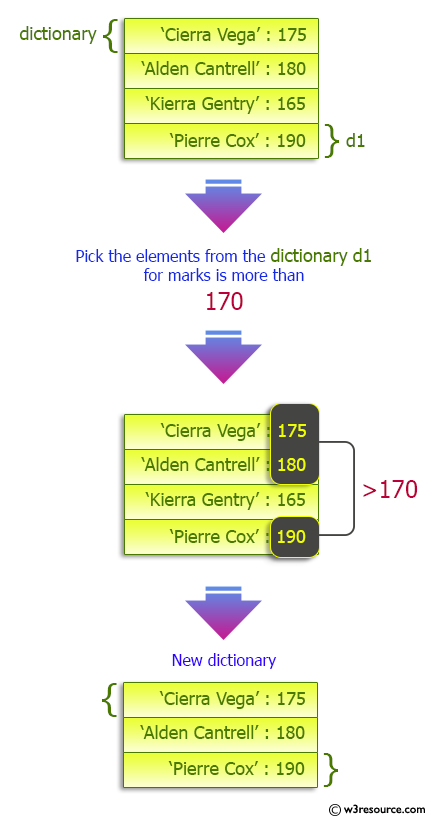
Sample Solution:
Python Code:
# Create a dictionary 'marks' with student names as keys and their marks as values.
marks = {'Cierra Vega': 175, 'Alden Cantrell': 180, 'Kierra Gentry': 165, 'Pierre Cox': 190}
# Print a message indicating the start of the code section and the original dictionary.
print("Original Dictionary:")
print(marks)
# Print a message indicating the intention to filter marks greater than or equal to 170.
print("Marks greater than 170:")
# Use a dictionary comprehension to create a new dictionary containing only the key-value pairs where marks are greater than or equal to 170.
# Iterate through the key-value pairs in 'marks' and include them in the new dictionary if the value is greater than or equal to 170.
result = {key: value for (key, value) in marks.items() if value >= 170}
# Print the new dictionary containing only the filtered key-value pairs.
print(result)
Sample Output:
Original Dictionary: {'Cierra Vega': 175, 'Alden Cantrell': 180, 'Kierra Gentry': 165, 'Pierre Cox': 190} Marks greater than 170: {'Cierra Vega': 175, 'Alden Cantrell': 180, 'Pierre Cox': 190}
Flowchart:
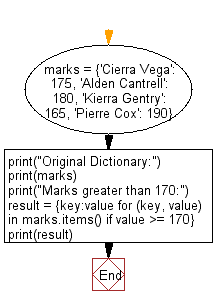
Python Code Editor:
Previous: Write a Python program to drop empty Items from a given Dictionary.
Next: Write a Python program to convert more than one list to nested dictionary.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics