Python: Check all values are same in a dictionary
Python dictionary: Exercise-45 with Solution
Write a Python program to verify that all values in a dictionary are the same.
Visual Presentation:
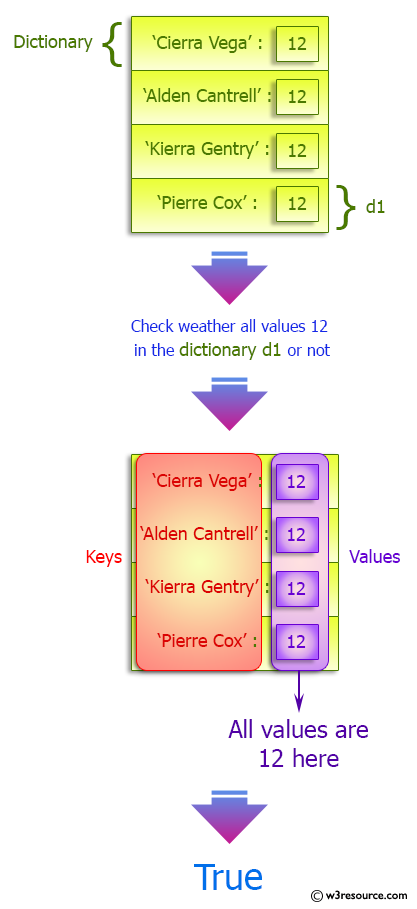
Sample Solution:
Python Code:
# Define a function 'value_check' that checks if all values in a dictionary are equal to a given value 'n'.
def value_check(students, n):
# Use the 'all' function to check if all values in the 'students' dictionary are equal to 'n'.
result = all(x == n for x in students.values())
return result
# Create a dictionary 'students' with student names as keys and values representing their scores.
students = {'Cierra Vega': 12, 'Alden Cantrell': 12, 'Kierra Gentry': 12, 'Pierre Cox': 12}
# Print a message indicating the start of the code section and the original dictionary.
print("Original Dictionary:")
print(students)
# Set the value 'n' to be checked to 12.
n = 12
# Print a message indicating the intention to check if all values in the dictionary are equal to 'n'.
print("\nCheck all are", n, "in the dictionary.")
# Call the 'value_check' function to check if all values in the dictionary are equal to 'n'.
# Print the result of the check.
print(value_check(students, n))
# Set the value 'n' to be checked to 10.
n = 10
# Print a message indicating the intention to check if all values in the dictionary are equal to 'n'.
print("\nCheck all are", n, "in the dictionary.")
# Call the 'value_check' function to check if all values in the dictionary are equal to 'n'.
# Print the result of the check.
print(value_check(students, n))
Sample Output:
Original Dictionary: {'Cierra Vega': 12, 'Alden Cantrell': 12, 'Kierra Gentry': 12, 'Pierre Cox': 12} Check all are 12 in the dictionary. True Check all are 10 in the dictionary. False
Flowchart:
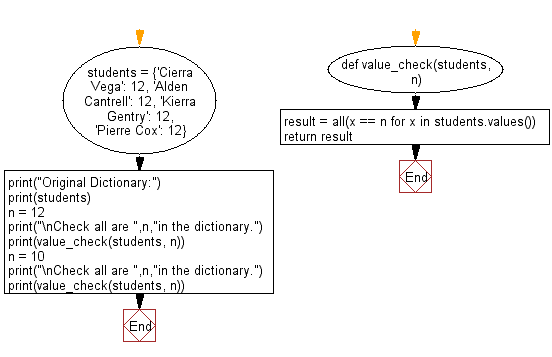
Python Code Editor:
Previous: Write a Python program to filter the height and width of students, which are stored in a dictionary.
Next: Write a Python program to create a dictionary grouping a sequence of key-value pairs into a dictionary of lists.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/dictionary/python-data-type-dictionary-exercise-45.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics