Python: Find the length of a given dictionary values
53. Find Length of a Dictionary
Write a Python program to find the length of a dictionary of values.
Visual Presentation:
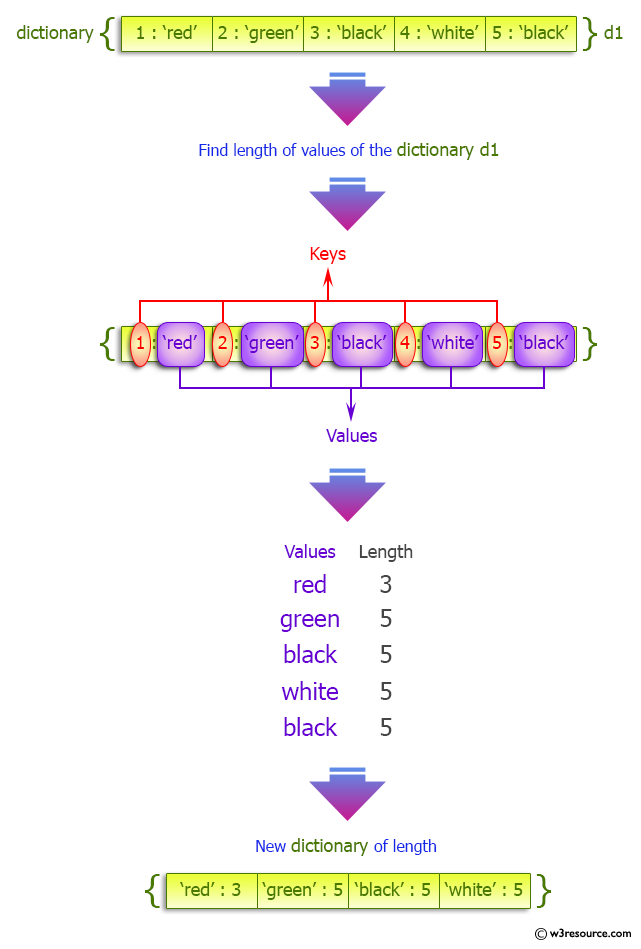
Sample Solution:
Python Code:
# Define a function 'test' that calculates the length of the values in a dictionary and returns a new dictionary with values as keys and their lengths as values.
def test(dictt):
result = {}
for val in dictt.values():
# Calculate the length of each value and store it as a key-value pair in the 'result' dictionary.
result[val] = len(val)
return result
# Create a dictionary 'color_dict' with integer keys and color values.
color_dict = {1: 'red', 2: 'green', 3: 'black', 4: 'white', 5: 'black'}
# Print a message indicating the start of the code section and the original dictionary.
print("\nOriginal Dictionary:")
print(color_dict)
# Print a message indicating the intention to calculate the length of dictionary values.
print("Length of dictionary values:")
# Call the 'test' function to calculate the length of values in the dictionary and print the result.
print(test(color_dict))
# Create a dictionary 'color_dict' with string keys and names as values.
color_dict = {'1': 'Austin Little', '2': 'Natasha Howard', '3': 'Alfred Mullins', '4': 'Jamie Rowe'}
# Print a message indicating the start of the code section and the original dictionary.
print("\nOriginal Dictionary:")
print(color_dict)
# Print a message indicating the intention to calculate the length of dictionary values.
print("Length of dictionary values:")
# Call the 'test' function to calculate the length of values in the dictionary and print the result.
print(test(color_dict))
Sample Output:
Original Dictionary: {1: 'red', 2: 'green', 3: 'black', 4: 'white', 5: 'black'} Length of dictionary values: {'red': 3, 'green': 5, 'black': 5, 'white': 5} Original Dictionary: {'1': 'Austin Little', '2': 'Natasha Howard', '3': 'Alfred Mullins', '4': 'Jamie Rowe'} Length of dictionary values: {'Austin Little': 13, 'Natasha Howard': 14, 'Alfred Mullins': 14, 'Jamie Rowe': 10}
Flowchart:
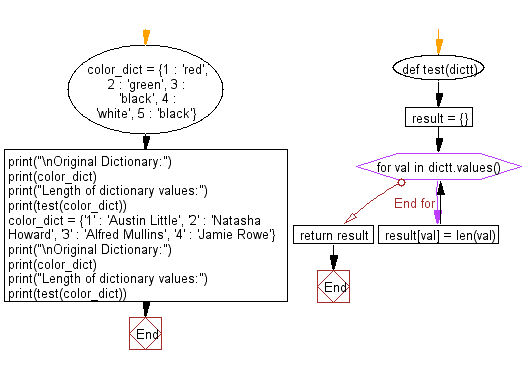
For more Practice: Solve these Related Problems:
- Write a Python program to determine the number of key-value pairs in a dictionary using the len() function.
- Write a Python program to iterate through a dictionary and manually count its items.
- Write a Python program to implement a recursive function that calculates the length of a dictionary.
- Write a Python program to use list comprehension on dictionary keys to compute the total count of entries.
Go to:
Previous: Write a Python program to extract a list of values from a given list of dictionaries.
Next:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.