Python: Combine two or more dictionaries, creating a list of values for each key
Python dictionary: Exercise-68 with Solution
Write a Python program to combine two or more dictionaries, creating a list of values for each key.
- Create a new collections.defaultdict with list as the default value for each key and loop over dicts.
- Use dict.append() to map the values of the dictionary to keys.
- Use dict() to convert the collections.defaultdict to a regular dictionary.
Sample Solution:
Python Code:
# Import the 'defaultdict' class from the 'collections' module.
from collections import defaultdict
# Define a function 'test' that takes any number of dictionaries as arguments (*dicts).
def test(*dicts):
# Create a 'defaultdict' object 'result' with a default value of an empty list.
result = defaultdict(list)
# Iterate through the dictionaries passed as arguments.
for el in dicts:
# Iterate through the keys and values in each dictionary.
for key in el:
# Append the value associated with the 'key' to the list for that 'key' in 'result'.
result[key].append(el[key])
# Convert the 'defaultdict' 'result' back to a regular dictionary and return it.
return dict(result)
# Define two dictionaries 'd1' and 'd2' with key-value pairs.
d1 = {'w': 50, 'x': 100, 'y': 'Green', 'z': 400}
d2 = {'x': 300, 'y': 'Red', 'z': 600}
# Print the original dictionaries.
print("Original dictionaries:")
print(d1)
print(d2)
# Call the 'test' function with 'd1' and 'd2' and print the combined dictionary.
print("\nCombined dictionaries, creating a list of values for each key:")
print(test(d1, d2))
Sample Output:
Original dictionaries: {'w': 50, 'x': 100, 'y': 'Green', 'z': 400} {'x': 300, 'y': 'Red', 'z': 600} Combined dictionaries, creating a list of values for each key: {'w': [50], 'x': [100, 300], 'y': ['Green', 'Red'], 'z': [400, 600]}
Flowchart:
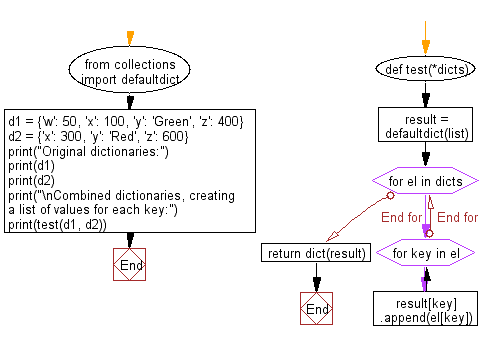
Python Code Editor:
Previous: Write a Python program to invert a given dictionary with non-unique hashable values.
Next: Write a Python program to group the elements of a given list based on the given function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/dictionary/python-data-type-dictionary-exercise-68.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics