Python frozenset symmetric difference: Function and example
3. Frozenset Symmetric Difference
Write a Python function that calculates the symmetric difference between two frozenset instances.
Sample Solution:
Code:
def symmetric_difference(frozenset_x, frozenset_y):
return frozenset_x.symmetric_difference(frozenset_y)
def main():
frozenset_a = frozenset([1, 2, 3, 4, 5, 6])
frozenset_b = frozenset([4, 5, 6, 7, 8])
print("Original frozensets:")
print(frozenset_a)
print(frozenset_b)
sym_diff_result = symmetric_difference(frozenset_a, frozenset_b)
print("\nSymmetric Difference of said two frozenset:", sym_diff_result)
if __name__ == "__main__":
main()
Output:
Original frozensets: frozenset({1, 2, 3, 4, 5, 6}) frozenset({4, 5, 6, 7, 8}) Symmetric Difference of said two frozenset: frozenset({1, 2, 3, 7, 8})
Flowchart:
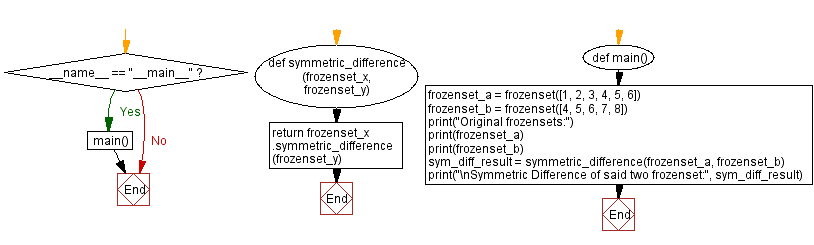
For more Practice: Solve these Related Problems:
- Write a Python function that computes the symmetric difference of two frozensets using the built-in operator and prints the result.
- Write a Python script to implement a custom algorithm for finding the symmetric difference of two frozensets and then verify it against the built-in method.
- Write a Python program to compare the symmetric difference of two frozensets with the union of their differences and print both outcomes.
- Write a Python function that takes two frozensets and returns True if their symmetric difference is empty, and False otherwise.
Go to:
Previous: Python frozen set and set conversion: Differences and comparisons.
Next: Python program: Generating power set of a frozenset.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.