Python: Find the nth ugly number using Heap queue algorithm
18. Nth Ugly Number
Write a Python program to find the nth ugly number using the heap queue algorithm.
Ugly numbers are positive numbers whose only prime factors are 2, 3 or 5. The sequence 1, 2, 3, 4, 5, 6, 8, 9, 10, 12, ... shows the first 10 ugly numbers.
Note: 1 is typically treated as an ugly number.
Sample Solution:
Python Code:
import heapq
class Solution(object):
#:type n: integer
#:return type: integer
def nth_Ugly_Number(self, n):
ugly_num = 0
heap = []
heapq.heappush(heap, 1)
for _ in range(n):
ugly_num = heapq.heappop(heap)
if ugly_num % 2 == 0:
heapq.heappush(heap, ugly_num * 2)
elif ugly_num % 3 == 0:
heapq.heappush(heap, ugly_num * 2)
heapq.heappush(heap, ugly_num * 3)
else:
heapq.heappush(heap, ugly_num * 2)
heapq.heappush(heap, ugly_num * 3)
heapq.heappush(heap, ugly_num * 5)
return ugly_num
n = 7
S = Solution()
result = S.nth_Ugly_Number(n)
print("7th Ugly number:")
print(result)
n = 10
result = S.nth_Ugly_Number(n)
print("\n10th Ugly number:")
print(result)
Sample Output:
7th Ugly number: 8 10th Ugly number: 12
Flowchart:
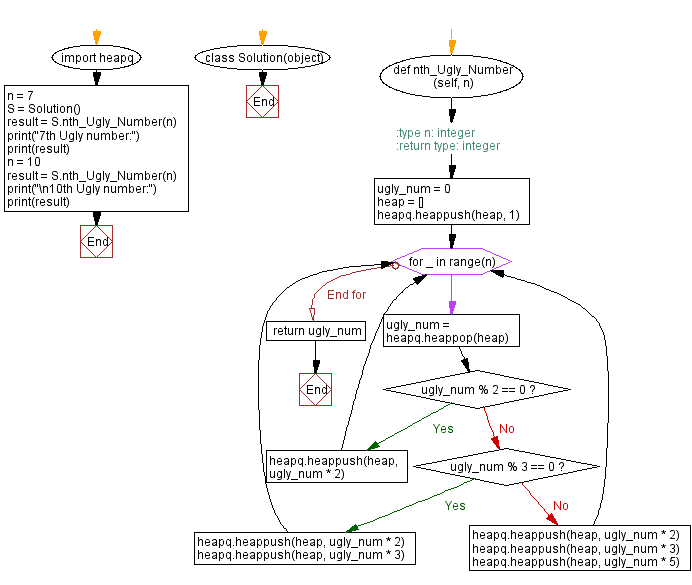
For more Practice: Solve these Related Problems:
- Write a Python program to compute the nth ugly number using a min-heap to generate ugly numbers from factors 2, 3, and 5.
- Write a Python script to find the nth ugly number by iteratively pushing multiples of 2, 3, and 5 onto a heap and then extracting the minimum.
- Write a Python program to implement a function that returns the nth ugly number using heapq while avoiding duplicates.
- Write a Python function to generate ugly numbers using a heap-based approach and then output the nth ugly number for different values of n.
Go to:
Previous: You are given two integer arrays sorted in ascending order and an integer k. Write a Python program to find k number of pairs (u, v) which consists of one element from the first array and one element from the second array using Heap queue algorithm.
Next: Write a Python program to print a heap as a tree-like data structure.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.