Python List Advanced Exercise - Find the union and intersection of two lists
Python List Advanced: Exercise-7 with Solution
Write a Python a function to find the union and intersection of two lists.
Sample Solution:
Python Code:
# Define a function to find the union and intersection of two lists
def union_intersection(lst1, lst2):
# Calculate the union of the two lists by converting them to sets and using the union operator '|'
union = list(set(lst1) | set(lst2))
# Calculate the intersection of the two lists by converting them to sets and using the intersection operator '&'
intersection = list(set(lst1) & set(lst2))
# Return both the union and intersection
return union, intersection
# Create two lists of numbers
nums1 = [1, 2, 3, 4, 5]
nums2 = [3, 4, 5, 6, 7, 8]
# Print the original lists
print("Original lists:")
print(nums1)
print(nums2)
# Call the union_intersection function with the two number lists and store the result in 'result'
result = union_intersection(nums1, nums2)
# Print the union of the two lists
print("\nUnion of said two lists:")
print(result[0])
# Print the intersection of the two lists
print("\nIntersection of said two lists:")
print(result[1])
# Create two lists of colors
colors1 = ["Red", "Green", "Blue"]
colors2 = ["Red", "White", "Pink", "Black"]
# Print the original lists of colors
print("Original lists:")
print(colors1)
print(colors2)
# Call the union_intersection function with the two color lists and store the result in 'result'
result = union_intersection(colors1, colors2)
# Print the union of the two color lists
print("\nUnion of said two lists:")
print(result[0])
# Print the intersection of the two color lists
print("\nIntersection of said two lists:")
print(result[1])
Sample Output:
Original lists: [1, 2, 3, 4, 5] [3, 4, 5, 6, 7, 8] Union of said two lists: [1, 2, 3, 4, 5, 6, 7, 8] Intersection of said two lists: [3, 4, 5] Original lists: ['Red', 'Green', 'Blue'] ['Red', 'White', 'Pink', 'Black'] Union of said two lists: ['Pink', 'Blue', 'White', 'Black', 'Red', 'Green'] Intersection of said two lists: ['Red']
Flowchart:
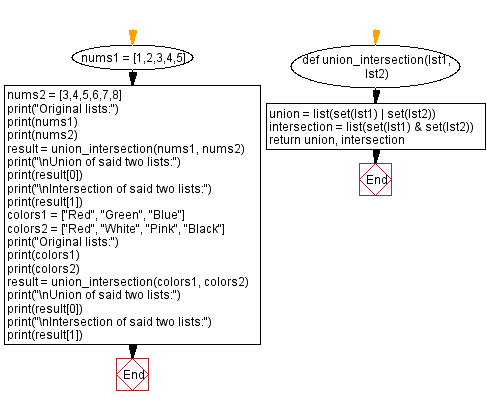
What is the time complexity and space complexity of the following Python code?
def union_intersection(lst1, lst2):
union = list(set(lst1) | set(lst2))
intersection = list(set(lst1) & set(lst2))
return union, intersection
Time complexity - The time complexity of the said code is O(n), where n is the combined length of "lst1" and "lst2". The set() function is used to remove duplicates from the input lists, which takes O(n) time in the worst case. The | and & operators are used to compute the union and intersection of the two sets "lst1" and "lst2", which also takes O(n) time in the worst case.
Space complexity – The space complexity of this code is also O(n), since two new lists "union" and "intersection" are created to store the union and intersection of the input lists "lst1" and "lst2". In addition, two sets (set(lst1) and set(lst2)) are also created to store the unique elements of "lst1" and "lst2". The space used by these sets depends on the number of unique elements in the input lists and is typically less than the total length of the lists. In the worst case, where there are no duplicates, the space complexity is O(n).
Python Code Editor:
Previous: Check if a list is a palindrome or not.
Next: Preserve order by removing duplicates.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/list-advanced/python-list-advanced-exercise-7.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics