Python: Reverse a given list of lists
Python List: Exercise - 150 with Solution
Reverse List of Lists
Write a Python program to reverse a given list of lists.
Sample Solution:
Python Code:
# Define a function called reverse_list_of_lists that takes a single argument 'list1'.
def reverse_list_of_lists(list1):
# Use slicing to reverse the order of the elements in the 'list1'.
return list1[::-1]
# Create a list 'colors' containing sublists.
colors = [['orange', 'red'], ['green', 'blue'], ['white', 'black', 'pink']]
# Print the original list.
print("Original list:")
print(colors)
# Print a message indicating that the list of lists is being reversed.
print("\nReverse said list of lists:")
# Call the 'reverse_list_of_lists' function to reverse the 'colors' list and print the result.
print(reverse_list_of_lists(colors))
# Create a list 'nums' containing sublists with different lengths.
nums = [[1, 2, 3, 4], [0, 2, 4, 5], [2, 3, 4, 2, 4]]
# Print a message indicating that the original list is being displayed.
print("\nOriginal list:")
print(nums)
# Print a message indicating that the list of lists is being reversed.
print("\nReverse said list of lists:")
# Call the 'reverse_list_of_lists' function to reverse the 'nums' list and print the result.
print(reverse_list_of_lists(nums))
Sample Output:
Original list: [['orange', 'red'], ['green', 'blue'], ['white', 'black', 'pink']] Reverse said list of lists: [['white', 'black', 'pink'], ['green', 'blue'], ['orange', 'red']] Original list: [[1, 2, 3, 4], [0, 2, 4, 5], [2, 3, 4, 2, 4]] Reverse said list of lists: [[2, 3, 4, 2, 4], [0, 2, 4, 5], [1, 2, 3, 4]]
Flowchart:
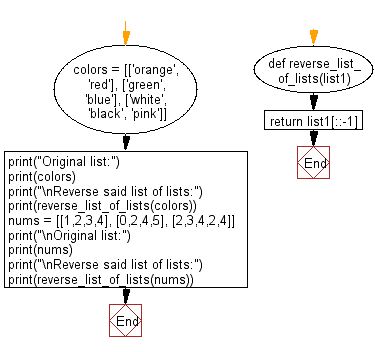
Python Code Editor:
Previous: Write a Python program to get all possible combinations of the elements of a given list.
Next: Write a Python program to find the maximum and minimum values in a given list within specified index range.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/list/python-data-type-list-exercise-150.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics