Python: Convert a given list of tuples to a list of strings
Convert Tuples to Strings
Write a Python program to convert a given list of tuples to a list of strings.
Visual Presentation:
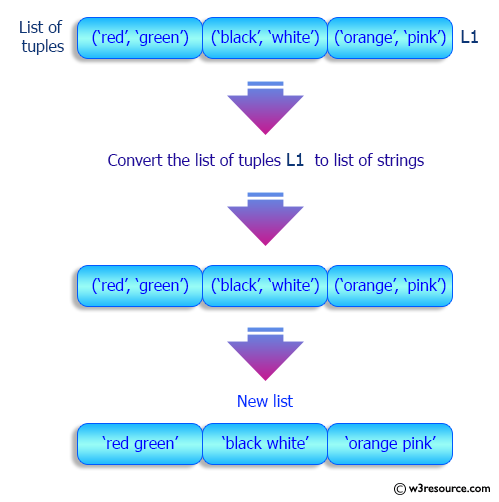
Sample Solution:
Python Code:
# Define a function called 'tuples_to_list_str' that converts a list of tuples to a list of strings.
def tuples_to_list_str(lst):
# Iterate through each tuple 'el' in the list 'lst'.
# Convert each element in the tuple to a string using the '%s' format specifier, and join them with spaces.
# Remove trailing spaces using 'strip'.
result = [("%s " * len(el) % el).strip() for el in lst]
return result
# Create a list of tuples 'colors', where each tuple contains color names.
colors = [('red', 'green'), ('black', 'white'), ('orange', 'pink')]
# Print a message indicating the original list of tuples.
print("Original list of tuples:")
# Print the original list 'colors'.
print(colors)
# Call the 'tuples_to_list_str' function with 'colors' and store the result.
result_colors = tuples_to_list_str(colors)
# Print a message indicating the conversion of the list of tuples to a list of strings.
print("\nConvert the said list of tuples to a list of strings:")
# Print the result 'result_colors'.
print(result_colors)
# Create a list of tuples 'names', where each tuple contains names.
names = [('Laiba', 'Delacruz'), ('Mali', 'Stacey', 'Drummond'), ('Raja', 'Welch'), ('Saarah', 'Stone')]
# Print a message indicating the original list of tuples.
print("\nOriginal list of tuples:")
# Print the original list 'names'.
print(names)
# Call the 'tuples_to_list_str' function with 'names' and store the result.
result_names = tuples_to_list_str(names)
# Print a message indicating the conversion of the list of tuples to a list of strings.
print("\nConvert the said list of tuples to a list of strings:")
# Print the result 'result_names'.
print(result_names)
Sample Output:
Original list of tuples: [('red', 'green'), ('black', 'white'), ('orange', 'pink')] Convert the said list of tuples to a list of strings: ['red green', 'black white', 'orange pink'] Original list of tuples: [('Laiba', 'Delacruz'), ('Mali', 'Stacey', 'Drummond'), ('Raja', 'Welch'), ('Saarah', 'Stone')] Convert the said list of tuples to a list of strings: ['Laiba Delacruz', 'Mali Stacey Drummond', 'Raja Welch', 'Saarah Stone']
Flowchart:
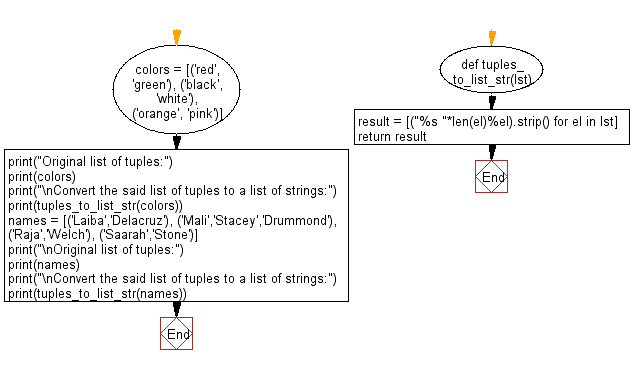
For more Practice: Solve these Related Problems:
- Write a Python program to convert a list of tuples to a list of strings by joining tuple elements with a hyphen.
- Write a Python program to convert a list of tuples to strings, handling non-string tuple elements appropriately.
- Write a Python program to convert a list of tuples to strings and then sort the resulting list alphabetically.
- Write a Python program to convert a list of tuples to formatted strings with each element capitalized.
Go to:
Previous: Write a Python program to swap two sublists in a given list.
Next: Write a Python program to sort a given list of tuples on specified element.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.