Python: Compute the average of nth elements in a given list of lists with different lengths
Python List: Exercise - 197 with Solution
Average N-th Element in Nested Lists
Write a Python program to compute the average of the n-th element in a given list of lists with different lengths.
Visual Presentation:
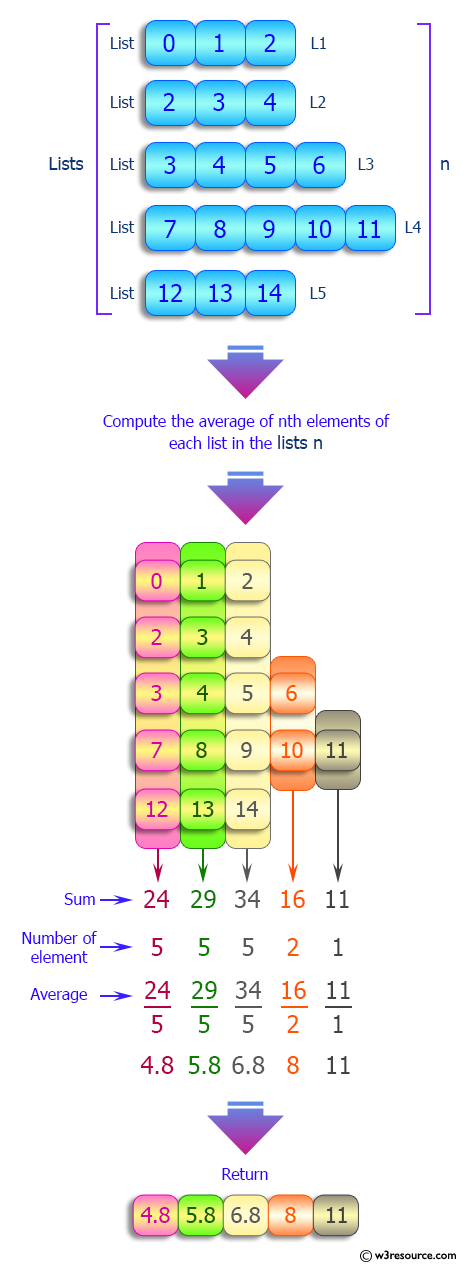
Sample Solution:
Python Code:
# Import the 'itertools' module as 'it' for convenience.
import itertools as it
# Create a list of lists 'nums' with varying lengths.
nums = [[0, 1, 2],
[2, 3, 4],
[3, 4, 5, 6],
[7, 8, 9, 10, 11],
[12, 13, 14]]
# Print a message indicating the original list of lists.
print("Original list:")
# Print the original 'nums' list.
print(nums)
# Define a function 'get_avg' that calculates the average of a list, ignoring 'None' values.
def get_avg(x):
# Remove 'None' values from the list 'x'.
x = [i for i in x if i is not None]
# Calculate the average of the remaining values.
return sum(x, 0.0) / len(x)
# Use the 'zip_longest' function from 'itertools' to iterate over the 'nums' lists and calculate the average.
result = map(get_avg, it.zip_longest(*nums))
# Print a message indicating the average of the n-th elements in the list of lists with different lengths.
print("\nAverage of n-th elements in the said list of lists with different lengths:")
# Convert the 'result' to a list and print it.
print(list(result))
Sample Output:
Original list: [[0, 1, 2], [2, 3, 4], [3, 4, 5, 6], [7, 8, 9, 10, 11], [12, 13, 14]] Average of n-th elements in the said list of lists with different lengths: [4.8, 5.8, 6.8, 8.0, 11.0]
Flowchart:
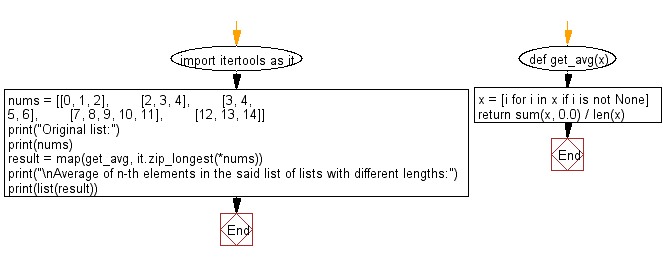
Python Code Editor:
Previous: Write a Python program to move a specified element in a given list.
Next: Write a Python program to compare two given lists and find the indices of the values present in both lists.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/list/python-data-type-list-exercise-197.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics