Python: Common tuples between two given lists
Python List: Exercise - 207 with Solution
Common Tuples in Two Lists
Write a Python program to find the common tuples between two given lists.
Visual Presentation:
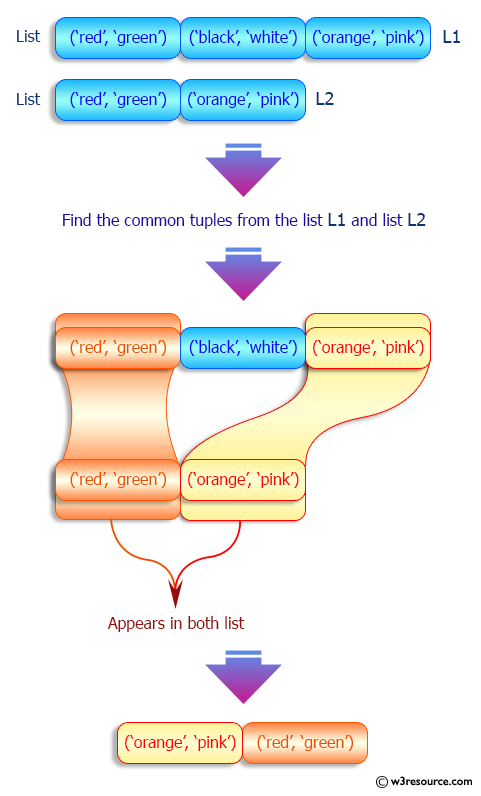
Sample Solution:
Python Code:
# Define a function 'test' that finds the common tuples between two lists of tuples.
def test(list1, list2):
# Convert 'list1' and 'list2' into sets to remove duplicate tuples and make intersection easier.
# Find the intersection (common tuples) between 'list1' and 'list2' using the 'intersection' method.
result = set(list1).intersection(list2)
# Convert the resulting set back to a list.
return list(result)
# Define two lists of tuples 'list1' and 'list2'.
list1 = [('red', 'green'), ('black', 'white'), ('orange', 'pink')]
list2 = [('red', 'green'), ('orange', 'pink')]
# Print a message indicating the original lists.
print("\nOriginal lists:")
# Print the original lists 'list1' and 'list2'.
print(list1)
print(list2)
# Print a message indicating the purpose of the following lines of code.
print("\nCommon tuples between two said lists")
# Call the 'test' function to find the common tuples between 'list1' and 'list2' and print the result.
print(test(list1, list2))
# Define two different lists of tuples 'list1' and 'list2'.
list1 = [('red', 'green'), ('orange', 'pink')]
list2 = [('red', 'green'), ('black', 'white'), ('orange', 'pink')]
# Print a message indicating the original lists.
print("\nOriginal lists:")
# Print the original lists 'list1' and 'list2'.
print(list1)
print(list2)
# Print a message indicating the purpose of the following lines of code.
print("\nCommon tuples between two said lists")
# Call the 'test' function to find the common tuples between 'list1' and 'list2' and print the result.
print(test(list1, list2))
Sample Output:
Original lists: [('red', 'green'), ('black', 'white'), ('orange', 'pink')] [('red', 'green'), ('orange', 'pink')] Common tuples between two said lists [('orange', 'pink'), ('red', 'green')] Original lists: [('red', 'green'), ('orange', 'pink')] [('red', 'green'), ('black', 'white'), ('orange', 'pink')] Common tuples between two said lists [('orange', 'pink'), ('red', 'green')]
Flowchart:
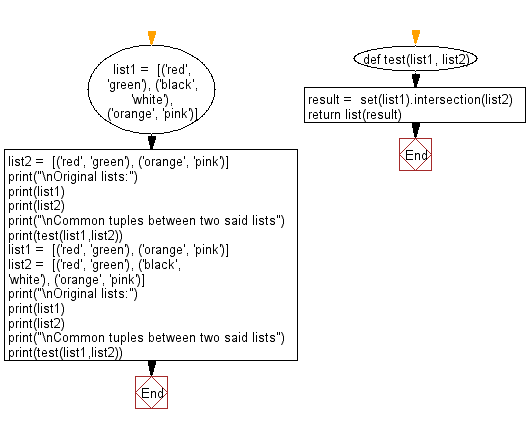
Python Code Editor:
Previous: Write a Python program to remove additional spaces in a given list.
Next: Sum a list of numbers. Write a Python program to sum the first number with the second and divide it by 2, then sum the second with the third and divide by 2, and so on.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/list/python-data-type-list-exercise-207.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics