Python: Sort one list based on another list containing the desired indexes
Sort List by Another List
Write a Python program to sort one list based on another list containing the desired indexes.
- Use zip() and sorted() to combine and sort the two lists, based on the values of indexes.
- Use a list comprehension to get the first element of each pair from the result
- Use the reverse parameter in sorted() to sort the dictionary in reverse order, based on the third argument
Sample Solution:
Python Code:
# Define a function called 'sort_by_indexes' that sorts a list based on a list of corresponding indexes.
def sort_by_indexes(lst, indexes, reverse=False):
# Use the 'sorted' function to sort the list 'lst' based on the corresponding indexes.
# The 'zip' function is used to pair each element of 'indexes' with the elements of 'lst'.
# The sorting is done based on the indexes, and the 'reverse' flag determines whether to sort in reverse order.
return [val for (_, val) in sorted(zip(indexes, lst), key=lambda x: x[0], reverse=reverse)]
# Example usage: Sort 'l1' based on the corresponding indexes in 'l2'.
l1 = ['eggs', 'bread', 'oranges', 'jam', 'apples', 'milk']
l2 = [3, 2, 6, 4, 1, 5]
print(sort_by_indexes(l1, l2)) # Sort in ascending order.
print(sort_by_indexes(l1, l2, True)) # Sort in descending order.
Sample Output:
['apples', 'bread', 'eggs', 'jam', 'milk', 'oranges'] ['oranges', 'milk', 'jam', 'eggs', 'bread', 'apples']
Flowchart:
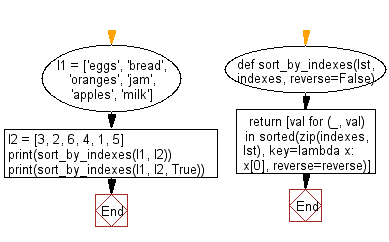
For more Practice: Solve these Related Problems:
- Write a Python program to reorder one list based on the sorted order of a second list.
- Write a Python program to sort a list of tuples by matching them with indices from another list.
- Write a Python program to sort a list using a custom order provided by a reference list.
- Write a Python program to rearrange a list based on a mapping of indices provided by another list.
Go to:
Previous: Write a Python program to split values into two groups, based on the result of the given filtering function.
Next: Write a Python program to build a list, using an iterator function and an initial seed value.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.