Python: Split values into two groups, based on the result of the given filter list
Python List: Exercise - 231 with Solution
Write a Python program to split values into two groups, based on the result of the given filter list.
- Use a list comprehension and zip() to add elements to groups, based on filter.
- If filter has a truthy value for any element, add it to the first group, otherwise add it to the second group.
Sample Solution:
Python Code:
# Define a function 'bifurcate' that takes a list 'colors' and a list of filters 'filter' as input.
def bifurcate(colors, filter):
# Use list comprehensions to create two lists: one with elements corresponding to True filter values and one with elements corresponding to False filter values.
return [
[x for x, flag in zip(colors, filter) if flag],
[x for x, flag in zip(colors, filter) if not flag]
]
# Call the 'bifurcate' function with example lists 'colors' and 'filter'.
print(bifurcate(['red', 'green', 'blue', 'pink'], [True, True, False, True]))
Sample Output:
[['red', 'green', 'pink'], ['blue']]
Flowchart:
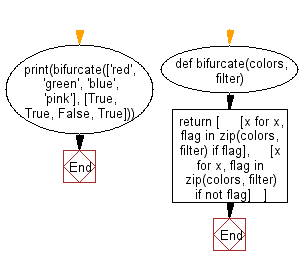
Python Code Editor:
Previous: Write a Python program to find the indexes of all elements in the given list that satisfy the provided testing function.
Next: Write a Python program to chunk a given list into smaller lists of a specified size.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics