Python: Create a dictionary with the unique values of a list as keys and their frequencies as the values
Frequency Dictionary from List
Write a Python program to create a dictionary with the unique values of a given list as keys and their frequencies as values.
- Use collections.defaultdict() to store the frequencies of each unique element.
- Use dict() to return a dictionary with the unique elements of the list as keys and their frequencies as the values.
Sample Solution:
Python Code:
# Import the 'defaultdict' class from the 'collections' module to create a dictionary with default values.
from collections import defaultdict
# Define a function 'frequencies' that takes a list 'lst' as input.
def frequencies(lst):
# Create an empty dictionary with default integer values.
freq = defaultdict(int)
# Iterate over the elements 'val' in the input list 'lst'.
for val in lst:
# Increase the count of the element 'val' in the dictionary by 1.
freq[val] += 1
# Convert the defaultdict to a regular dictionary and return the result.
return dict(freq)
# Call the 'frequencies' function with a list of strings to count the frequency of each string.
# Print the result.
print(frequencies(['a', 'b', 'f', 'a', 'c', 'e', 'a', 'a', 'b', 'e', 'f']))
# Call the 'frequencies' function again with a list of integers to count the frequency of each integer.
# Print the result.
print(frequencies([3, 4, 7, 5, 9, 3, 4, 5, 0, 3, 2, 3]))
Sample Output:
{'a': 4, 'b': 2, 'f': 2, 'c': 1, 'e': 2} {3: 4, 4: 2, 7: 1, 5: 2, 9: 1, 0: 1, 2: 1}
Flowchart:
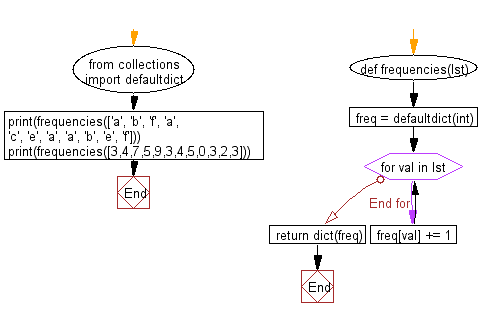
For more Practice: Solve these Related Problems:
- Write a Python program to create a frequency dictionary from a list of words, ignoring case and punctuation.
- Write a Python program to generate a frequency dictionary from a list of numbers and then filter out keys that occur only once.
- Write a Python program to compute the frequency dictionary of a list containing mixed data types, ensuring type-specific counting.
- Write a Python program to build a frequency dictionary from a list and then output the most and least frequent elements.
Go to:
Previous: Write a Python program to find the value of the last element in the given list that satisfies the provided testing function.
Next: Write a Python program to get the symmetric difference between two iterables, without filtering out duplicate values.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.