Python: Minimum value of a list, after mapping each element to a value using a giving function
Python List: Exercise - 249 with Solution
Min Mapped Value in List
Write a Python program to get the minimum value of a list, after mapping each element to a value using a given function.
- Use map() with fn to map each element to a value using the provided function.
- Use min() to return the minimum value.
Sample Solution:
Python Code:
# Define a function 'min_by' that takes a list 'lst' and a function 'fn'.
# It uses the 'min' function to find the minimum value in 'lst' based on the results of applying 'fn' to each element.
def min_by(lst, fn):
return min(map(fn, lst))
# Call the 'min_by' function with a list of dictionaries and a lambda function.
# The lambda function extracts the 'n' value from each dictionary.
# The function returns the minimum 'n' value from the list of dictionaries.
print(min_by([{ 'n': 4 }, { 'n': 2 }, { 'n': 8 }, { 'n': 6 }], lambda v : v['n']))
Sample Output:
2
Flowchart:
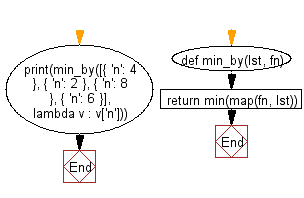
Python Code Editor:
Previous: Write a Python program to get the maximum value of a list, after mapping each element to a value using a given function.
Next: Write a Python program to calculate the sum of a list, after mapping each element to a value using the provided function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/list/python-data-type-list-exercise-249.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics