Python: Generate a list, containing the Fibonacci sequence, up until the nth term
Generate Fibonacci Sequence
Write a Python program to generate a list containing the Fibonacci sequence, up until the nth term.
- Starting with 0 and 1, use list.append() to add the sum of the last two numbers of the list to the end of the list, until the length of the list reaches n.
- If n is less or equal to 0, return a list containing 0.
Sample Solution:
Python Code:
# Define a function named 'fibonacci_nums' that generates a list of Fibonacci numbers up to the nth value.
def fibonacci_nums(n):
# Check if n is non-positive (less than or equal to 0).
if n <= 0:
# Return a list containing only 0 when n is not positive.
return [0]
# Initialize the Fibonacci sequence with the first two values, 0 and 1.
sequence = [0, 1]
# Continue generating the sequence until it reaches the desired length (n).
while len(sequence) <= n:
# Calculate the next value by adding the last two values in the sequence.
next_value = sequence[len(sequence) - 1] + sequence[len(sequence) - 2]
# Append the next value to the sequence.
sequence.append(next_value)
# Return the list of Fibonacci numbers.
return sequence
# Print the first 7 Fibonacci numbers.
print("First 7 Fibonacci numbers:")
print(fibonacci_nums(7))
# Print the first 15 Fibonacci numbers.
print("\nFirst 15 Fibonacci numbers:")
print(fibonacci_nums(15))
# Print the first 50 Fibonacci numbers (for demonstration purposes).
print("\nFirst 50 Fibonacci numbers:")
print(fibonacci_nums(50))
Sample Output:
First 7 Fibonacci numbers: [0, 1, 1, 2, 3, 5, 8, 13] First 15 Fibonacci numbers: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610] First 50 Fibonacci numbers: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610, 987, 1597, 2584, 4181, 6765, 10946, 17711, 28657, 46368, 75025, 121393, 196418, 317811, 514229, 832040, 1346269, 2178309, 3524578, 5702887, 9227465, 14930352, 24157817, 39088169, 63245986, 102334155, 165580141, 267914296, 433494437, 701408733, 1134903170, 1836311903, 2971215073, 4807526976, 7778742049, 12586269025]
Flowchart:
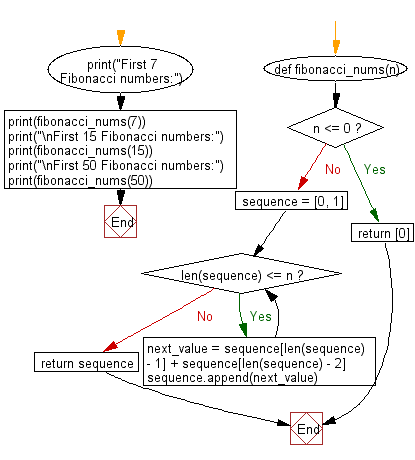
For more Practice: Solve these Related Problems:
- Write a Python program to generate Fibonacci numbers up to a given maximum value instead of a fixed term count.
- Write a Python program to generate the first n Fibonacci numbers recursively.
- Write a Python program to generate the Fibonacci sequence and then return the sequence in reverse order.
- Write a Python program to compute the sum of the first n Fibonacci numbers.
Go to:
Previous: Write a Python program to create a two-dimensional list from given list of lists.
Next: Write a Python program to cast the provided value as a list if it's not one.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.