Python Exercises: Largest, lowest gap between sorted values of a list
Largest and Smallest Gaps
Write a Python program to calculate the largest and smallest gap between sorted elements of a list of integers.
Sample Data:
{1, 2 ,9, 0, 4, 6} -> 3
{23, -2, 45, 38, 12, 4, 6} -> 15
Sample Solution-1:
Python Code:
# Define a function named 'test' that takes a list 'nums' as input.
def test(nums):
# Sort the elements in the input list 'nums' in ascending order.
nums.sort()
# Calculate the largest gap between consecutive sorted values and store it in 'max_gap'.
max_gap = max(b - a for a, b in zip(nums, nums[1:]))
# Calculate the smallest gap between consecutive sorted values and store it in 'min_gap'.
min_gap = min(b - a for a, b in zip(nums, nums[1:]))
# Return a tuple containing both the 'max_gap' and 'min_gap'.
return max_gap, min_gap
# Define the original list 'nums'.
nums = [0, 9, 2, 4, 5, 6]
print("Original list:")
print(nums)
# Call the 'test' function to find the largest and smallest gap between sorted values in the list.
print("Largest, lowest gap between sorted values of the said list:", test(nums))
# Define another list 'nums'.
nums = [23, -2, 45, 38, 12, 4, 6]
print("\nOriginal list:")
print(nums)
# Call the 'test' function for the second list of numbers.
print("Largest, lowest gap between sorted values of the said list:", test(nums))
# Define another list 'nums'.
nums = [1, 2, 3]
print("\nOriginal list:")
print(nums)
# Call the 'test' function for the third list of numbers.
print("Largest, lowest gap between sorted values of the said list:", test(nums))
# Define another list 'nums'.
nums = [-4, -3, 5, 20, 10, 1]
print("\nOriginal list:")
print(nums)
# Call the 'test' function for the fourth list of numbers.
print("Largest, lowest gap between sorted values of the said list:", test(nums))
Sample Output:
Original list: [0, 9, 2, 4, 5, 6] Largest, lowest gap between sorted values of the said list: (3, 1) Original list: [23, -2, 45, 38, 12, 4, 6] Largest, lowest gap between sorted values of the said list: (15, 2) Original list: [1, 2, 3] Largest, lowest gap between sorted values of the said list: (1, 1) Original list: [-4, -3, 5, 20, 10, 1] Largest, lowest gap between sorted values of the said list: (10, 1)
Flowchart:
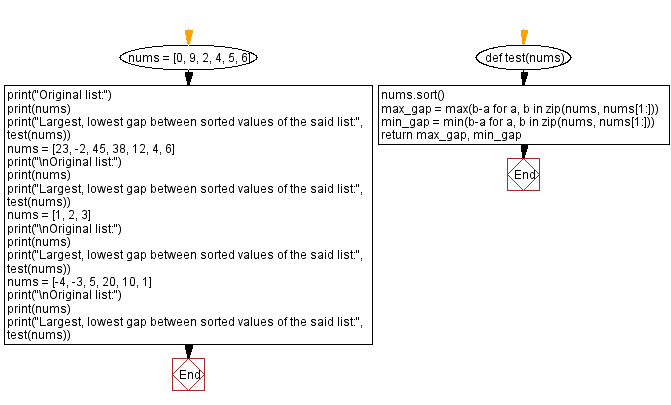
Sample Solution-2:
Python Code:
# Define a function named 'test' that takes a list 'nums' as input.
def test(nums):
# Sort the elements in the input list 'nums' in ascending order.
nums.sort()
# Create a list comprehension to calculate the differences between consecutive sorted values.
# Find the maximum difference in the sorted values and store it in 'max_gap'.
max_gap = max([sorted(nums)[i] - sorted(nums)[i-1] for i in range(1, len(nums))])
# Find the minimum difference in the sorted values and store it in 'min_gap'.
min_gap = min([sorted(nums)[i] - sorted(nums)[i-1] for i in range(1, len(nums))])
# Return a tuple containing both the 'max_gap' and 'min_gap'.
return max_gap, min_gap
# Define the original list 'nums'.
nums = [0, 9, 2, 4, 5, 6]
print("Original list:")
print(nums)
# Call the 'test' function to find the largest and smallest gap between sorted values in the list.
print("Largest, lowest gap between sorted values of the said list:", test(nums))
# Define another list 'nums'.
nums = [23, -2, 45, 38, 12, 4, 6]
print("\nOriginal list:")
print(nums)
# Call the 'test' function for the second list of numbers.
print("Largest, lowest gap between sorted values of the said list:", test(nums))
# Define another list 'nums'.
nums = [1, 2, 3]
print("\nOriginal list:")
print(nums)
# Call the 'test' function for the third list of numbers.
print("Largest, lowest gap between sorted values of the said list:", test(nums))
# Define another list 'nums'.
nums = [-4, -3, 5, 20, 10, 1]
print("\nOriginal list:")
print(nums)
# Call the 'test' function for the fourth list of numbers.
print("Largest, lowest gap between sorted values of the said list:", test(nums))
Sample Output:
Original list: [0, 9, 2, 4, 5, 6] Largest, lowest gap between sorted values of the said list: (3, 1) Original list: [23, -2, 45, 38, 12, 4, 6] Largest, lowest gap between sorted values of the said list: (15, 2) Original list: [1, 2, 3] Largest, lowest gap between sorted values of the said list: (1, 1) Original list: [-4, -3, 5, 20, 10, 1] Largest, lowest gap between sorted values of the said list: (10, 1)
Flowchart:
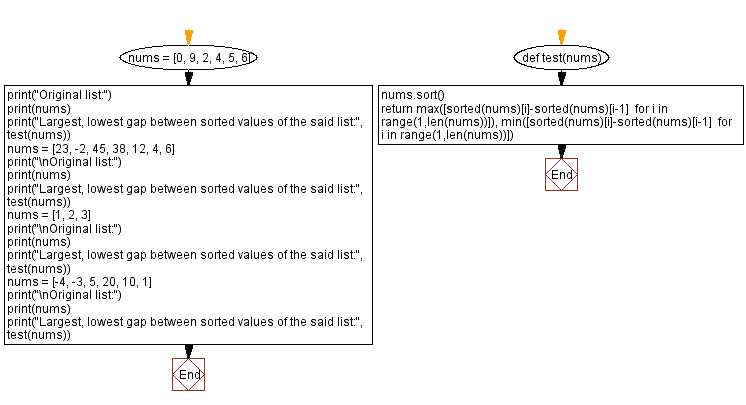
For more Practice: Solve these Related Problems:
- Write a Python program to calculate the largest gap between consecutive elements in a sorted list.
- Write a Python program to compute both the maximum and minimum gaps between adjacent sorted elements and return them as a tuple.
- Write a Python program to determine the average gap between sorted elements in a list of integers.
- Write a Python program to find the most frequently occurring gap between consecutive numbers in a sorted list.
Go to:
Previous Python Exercise: Find the largest odd number in a list of integers.
Next Python Exercise: Sum of missing numbers of a list of integers.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.