Python: Split a list based on first character of word
Split List by First Character
Write a Python program to split a list based on the first character of a word.
Sample Input:
word_list = ['be','have','do','say','get','make','go','know','take','see','come','think', 'look','want','give','use','find','tell','ask','work','seem','feel','leave','call']
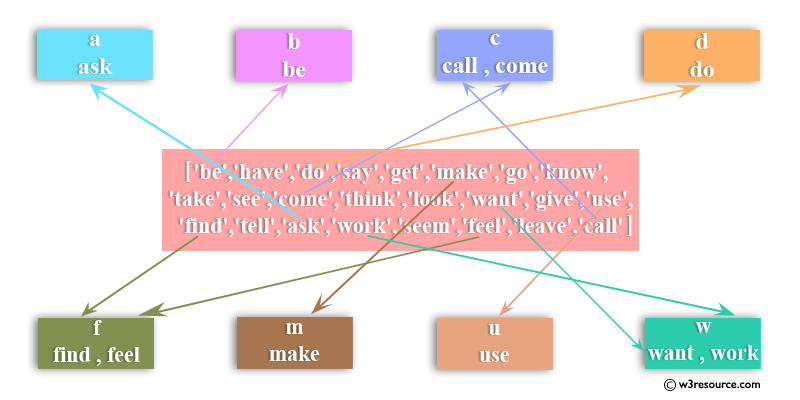
Sample Solution:
Python Code:
# Import the 'groupby' function from the 'itertools' module and the 'itemgetter' function from the 'operator' module
from itertools import groupby
from operator import itemgetter
# Define a list 'word_list' containing words
word_list = ['be', 'have', 'do', 'say', 'get', 'make', 'go', 'know', 'take', 'see', 'come', 'think',
'look', 'want', 'give', 'use', 'find', 'tell', 'ask', 'work', 'seem', 'feel', 'leave', 'call']
# Use 'groupby' to group and sort the words in 'word_list' based on the first letter of each word
# Iterate through the groups and print the first letter and the words starting with that letter
for letter, words in groupby(sorted(word_list), key=itemgetter(0)):
print(letter)
for word in words:
print(word)
Sample Output:
a ask b be c call come d do ----- w want work
Flowchart:
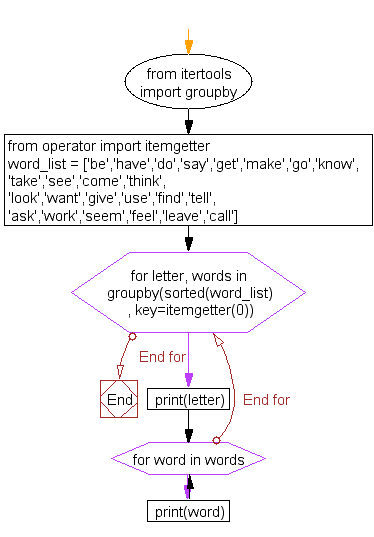
For more Practice: Solve these Related Problems:
- Write a Python program to group words in a list based on their first letter.
- Write a Python program to split a list into multiple lists based on the first character of each element.
- Write a Python program to sort a list of words and then group them by their first letter.
- Write a Python program to split a list of email addresses based on the first letter of the domain.
Go to:
Previous: Write a Python program to convert a list of multiple integers into a single integer.
Next: Write a Python program to create multiple lists.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.