Python: Convert list to list of dictionaries
Python List: Exercise - 49 with Solution
Write a Python program to convert a list to a list of dictionaries.
Sample Solution:
Python Code:
# Define a list 'color_name' containing color names
color_name = ["Black", "Red", "Maroon", "Yellow"]
# Define a list 'color_code' containing color codes
color_code = ["#000000", "#FF0000", "#800000", "#FFFF00"]
# Use a list comprehension to create a list of dictionaries by pairing elements from 'color_name' and 'color_code' using the 'zip' function
# Each dictionary has keys 'color_name' and 'color_code', and the values are taken from 'color_name' and 'color_code'
# This results in a list of dictionaries, where each dictionary represents a color with its name and code
# Print the list of color dictionaries
print([{'color_name': f, 'color_code': c} for f, c in zip(color_name, color_code)])
Sample Output:
[{'color_name': 'Black', 'color_code': '#000000'}, {'color_name': 'Red', 'color_code': '#FF0000'}, {'color_nam e': 'Maroon', 'color_code': '#800000'}, {'color_name': 'Yellow', 'color_code': '#FFFF00'}]
Flowchart:
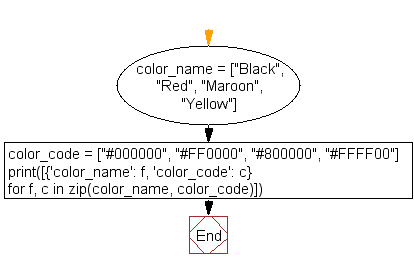
Python Code Editor:
Previous: Write a Python program to print a nested lists (each list on a new line) using the print() function.
Next: Write a Python program to sort a list of nested dictionaries.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics