Python Math: Print the floating point from mantissa, exponent pair
58. Mantissa and Exponent Printer
Write a Python program to print the floating point of the mantissa, exponent pair.
Sample Solution:
Python Code:
import math
print('{:^7} {:^8} {:^17}'.format('Mantissa', 'Exponent', 'Floating point value'))
print('{:-^8} {:-^8} {:-^20}'.format('', '', ''))
for m, e in [ (0.7, -3),
(0.3, 0),
(0.5, 3),
]:
x = math.ldexp(m, e)
print('{:7.2f} {:7d} {:7.2f}'.format(m, e, x))
Sample Output:
Mantissa Exponent Floating point value -------- -------- -------------------- 0.70 -3 0.09 0.30 0 0.30 0.50 3 4.00
Flowchart:
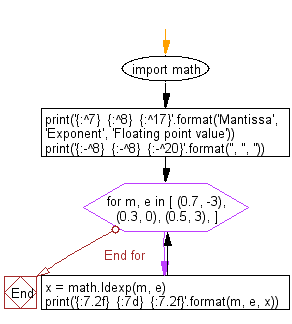
For more Practice: Solve these Related Problems:
- Write a Python program to use math.frexp() to decompose a floating-point number into its mantissa and exponent, and print them.
- Write a Python function that takes a float, retrieves its mantissa and exponent, and returns a formatted string displaying both values.
- Write a Python script to iterate over a list of floating-point numbers, print each number’s mantissa and exponent, and then convert them back to the original number.
- Write a Python program to display the mantissa and exponent of several floating-point values in a tabulated format using math.frexp().
Go to:
Previous: Write a Python program to calculate the standard deviation of the following data.
Next: Write a Python program to split fractional and integer parts of a floating point number.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.