Python: Create a deep copy of a given dictionary
4. Deep Copy of Dictionary
Write a Python program to create a deep copy of a given dictionary. Use copy.copy
Sample Solution:
Python Code:
import copy
nums_x = {"a":1, "b":2, 'cc':{"c":3}}
print("Original dictionary: ", nums_x)
nums_y = copy.deepcopy(nums_x)
print("\nDeep copy of the said list:")
print(nums_y)
print("\nChange the value of an element of the original dictionary:")
nums_x["cc"]["c"] = 10
print(nums_x)
print("\nSecond dictionary (Deep copy):")
print(nums_y)
nums = {"x":1, "y":2, 'zz':{"z":3}}
nums_copy = copy.deepcopy(nums)
print("\nOriginal dictionary :")
print(nums)
print("\nDeep copy of the said list:")
print(nums_copy)
print("\nChange the value of an element of the original dictionary:")
nums["zz"]["z"] = 10
print("\nFirst dictionary:")
print(nums)
print("\nSecond dictionary (Deep copy):")
print(nums_copy)
Sample Output:
Original dictionary: {'a': 1, 'b': 2, 'cc': {'c': 3}} Deep copy of the said list: {'a': 1, 'b': 2, 'cc': {'c': 3}} Change the value of an element of the original dictionary: {'a': 1, 'b': 2, 'cc': {'c': 10}} Second dictionary (Deep copy): {'a': 1, 'b': 2, 'cc': {'c': 3}} Original dictionary : {'x': 1, 'y': 2, 'zz': {'z': 3}} Deep copy of the said list: {'x': 1, 'y': 2, 'zz': {'z': 3}} Change the value of an element of the original dictionary: First dictionary: {'x': 1, 'y': 2, 'zz': {'z': 10}} Second dictionary (Deep copy): {'x': 1, 'y': 2, 'zz': {'z': 3}}
Flowchart:
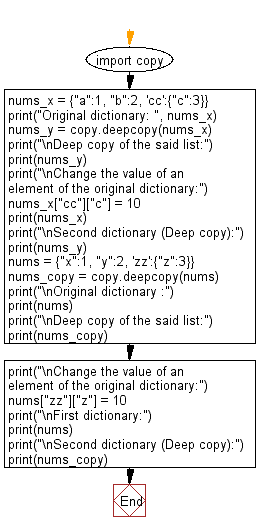
For more Practice: Solve these Related Problems:
- Write a Python program to create a deep copy of a dictionary with nested lists using copy.deepcopy, then modify the nested list in the original and print both dictionaries to verify independence.
- Write a Python function that accepts a dictionary with nested structures, makes a deep copy using copy.deepcopy, and then compares the id() of nested objects between the original and the copy.
- Write a Python script to demonstrate the deep copy process by updating a nested value in a dictionary after performing a deep copy, ensuring that the change does not affect the copy.
- Write a Python program to convert a complex dictionary to a deep copy using copy.deepcopy and then output both the original and copied dictionaries to confirm that no shared mutable objects exist.
Go to:
Previous: Write a Python program to create a shallow copy of a given dictionary.
Next: Python built-in Modules Exercise Home.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.