Python: Construct a Decimal from a float and a Decimal from a string
1. Decimal Construction
Write a Python program to construct a Decimal from a float and a Decimal from a string. Also represent the decimal value as a tuple. Use decimal.Decimal
Sample Solution:
Python Code:
import decimal
print("Construct a Decimal from a float:")
pi_val = decimal.Decimal(3.14159)
print(pi_val)
print(pi_val.as_tuple())
print("\nConstruct a Decimal from a string:")
num_str = decimal.Decimal("123.25")
print(num_str)
print(num_str.as_tuple())
Sample Output:
Construct a Decimal from a float: 3.14158999999999988261834005243144929409027099609375 DecimalTuple(sign=0, digits=(3, 1, 4, 1, 5, 8, 9, 9, 9, 9, 9, 9, 9, 9, 9, 9, 8, 8, 2, 6, 1, 8, 3, 4, 0, 0, 5, 2, 4, 3, 1, 4, 4, 9, 2, 9, 4, 0, 9, 0, 2, 7, 0, 9, 9, 6, 0, 9, 3, 7, 5), exponent=-50) Construct a Decimal from a string: 123.25 DecimalTuple(sign=0, digits=(1, 2, 3, 2, 5), exponent=-2)
Flowchart:
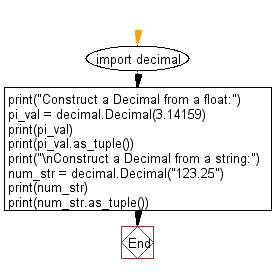
For more Practice: Solve these Related Problems:
- Write a Python program to construct a Decimal from a float, a Decimal from a string, and then represent both as tuples using the as_tuple() method.
- Write a Python function that takes a float and a string, converts them into Decimal objects, and prints their tuple representations side by side.
- Write a Python script to generate a Decimal from a float and one from a string, compare their tuple representations, and print whether they are identical.
- Write a Python program that converts a list of floats and a list of corresponding strings to Decimals, then prints each Decimal’s tuple representation.
Go to:
Previous: Python module Exercises Home.
Next: Write a Python program to configure the rounding to round up and round down a given decimal value.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.