Python: Construct a seeded random number and float number generator
Python module: Exercise-4 with Solution
Write a Python program to construct a seeded random number generator, also generate a float between 0 and 1, excluding 1.
Use random.random()
Sample Solution:
Python Code:
import random
print("Construct a seeded random number generator:")
print(random.Random().random())
print(random.Random(0).random())
print("\nGenerate a float between 0 and 1, excluding 1:")
print(random.random())
Sample Output:
Construct a seeded random number generator: 0.4209937412831368 0.8444218515250481 Generate a float between 0 and 1, excluding 1: 0.5000494615698478
Flowchart:
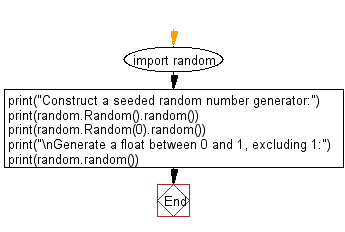
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to generate a random alphabetical character, alphabetical string and alphabetical string of a fixed length.
Next: Write a Python program to generate a random integer between 0 and 6 - excluding 6, random integer between 5 and 10 - excluding 10, random integer between 0 and 10, with a step of 3 and random date between two dates.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics