NumPy: Generate a random number between 0 and 1
NumPy: Basic Exercise-17 with Solution
Generate Random Number [0,1]
Write a NumPy program to generate a random number between 0 and 1.
This NumPy program generates a random number between 0 and 1. By utilizing NumPy's random number generation functions, it efficiently produces a floating-point number within the specified range. This program demonstrates the ease of generating random values using NumPy's built-in capabilities.
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Generating a random number from a normal distribution with mean 0 and standard deviation 1 using np.random.normal()
rand_num = np.random.normal(0, 1, 1)
# Printing a message indicating a random number between 0 and 1
print("Random number between 0 and 1:")
# Printing the generated random number
print(rand_num)
Output:
Random number between 0 and 1: [-1.15262276]
Explanation:
In the said code -
The np.random.normal() function generates a random number from a normal distribution with a mean (loc) of 0 and a standard deviation (scale) of 1. The third argument, 1, specifies the number of random numbers to generate, which is a single number in this case.
Finally print(rand_num) prints the generated random number to the console. The output will vary each time the code is executed due to the random nature of the function.
Visual Presentation:
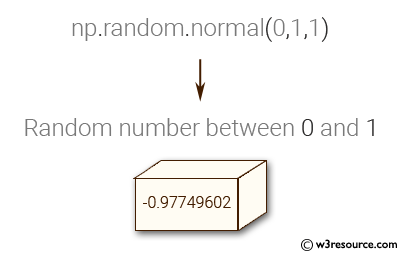
Python-Numpy Code Editor:
Previous: NumPy program to create a 3x3 identity matrix.
Next: NumPy program to generate an array of 15 random numbers from a standard normal distribution.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/numpy/basic/numpy-basic-exercise-17.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics