NumPy: Create a vector with values from 0 to 20 and change the sign of the numbers in the range from 9 to 15
NumPy: Basic Exercise-22 with Solution
Change Sign of Range 9-15
Write a NumPy program to create a vector with values from 0 to 20 and change the sign of the numbers in the range from 9 to 15.
The problem involves writing a NumPy program to generate a vector ranging from 0 to 20, with specific values modified. Specifically, the task requires changing the sign of numbers within the range of 9 to 15 while leaving the rest of the values unchanged. This involves leveraging NumPy's array manipulation capabilities to efficiently achieve the desired outcome.
Sample Solution :
Python Code :
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a NumPy array 'x' containing integers from 0 to 20 using np.arange()
x = np.arange(21)
# Printing a message indicating the original vector 'x'
print("Original vector:")
print(x)
# Printing a message indicating changing the sign of numbers in the range from 9 to 15
# Using boolean indexing, multiplying elements between 9 and 15 by -1 to change their sign
x[(x >= 9) & (x <= 15)] *= -1
# Printing the modified vector 'x'
print("After changing the sign of the numbers in the range from 9 to 15:")
print(x)
Output:
Original vector: [ 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20] After changing the sign of the numbers in the range from 9 to 15: [ 0 1 2 3 4 5 6 7 8 -9 -10 -11 -12 -13 -14 -15 16 17 18 19 20]
Explanation:
The above code creates an array of integers from 0 to 20 and negates the elements with values between 9 and 15 (inclusive).
At first the np.arange() function creates a NumPy array 'x' containing integers from 0 to 20.
Secondly x[(x >= 9) & (x <= 15)] *= -1 uses boolean indexing to modify a specific subset of elements in the array 'x'. The condition (x >= 9) & (x <= 15) creates a boolean mask that is True for elements with values between 9 and 15 (inclusive), and False for all other elements. The mask is then applied to the array 'x', and the selected elements (those with True values in the mask) are multiplied by -1, effectively negating them.
Finally print(x) prints the modified array to the console.Visual Presentation:
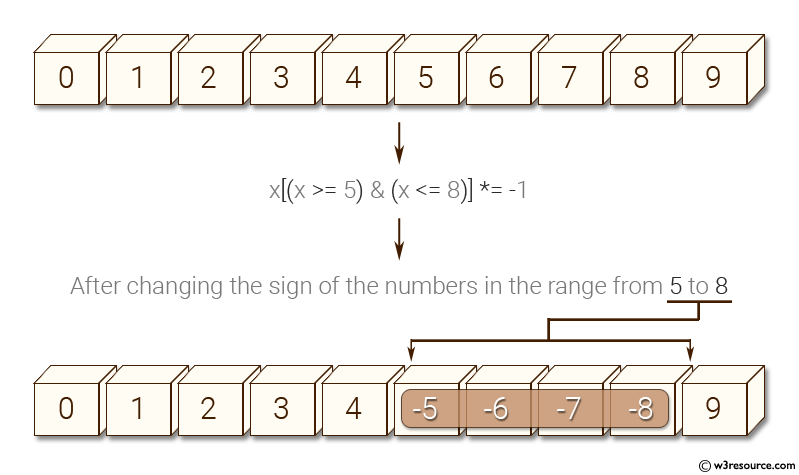
Python-Numpy Code Editor:
Previous: NumPy program to create a vector of length 10 with values evenly distributed between 5 and 50.
Next: NumPy program to create a vector of length 5 filled with arbitrary integers from 0 to 10.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/numpy/basic/numpy-basic-exercise-22.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics