NumPy: Find the set difference of two arrays
Set Difference of Arrays
Write a NumPy program to find the set difference between two arrays. The set difference will return sorted, distinct values in array1 that are not in array2.
Pictorial Presentation:
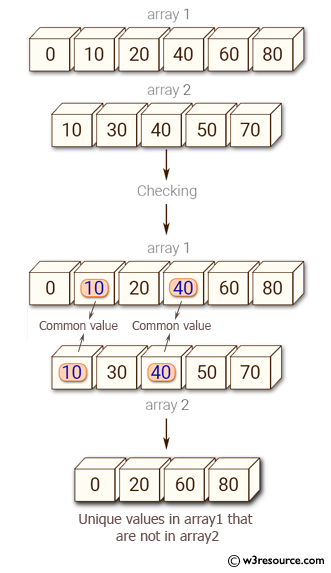
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a NumPy array 'array1'
array1 = np.array([0, 10, 20, 40, 60, 80])
# Printing the contents of 'array1'
print("Array1: ", array1)
# Creating a Python list 'array2'
array2 = [10, 30, 40, 50, 70]
# Printing the contents of 'array2'
print("Array2: ", array2)
# Finding and printing the unique values in 'array1' that are not present in 'array2'
print("Unique values in array1 that are not in array2:")
print(np.setdiff1d(array1, array2))
Sample Output:
Array1: [ 0 10 20 40 60 80] Array2: [10, 30, 40, 50, 70] Unique values in array1 that are not in array2: [ 0 20 60 80]
Explanation:
In the above code -
array1 = np.array([0, 10, 20, 40, 60, 80]): Creates a NumPy array with elements 0, 10, 20, 40, 60, and 80.
array2 = [10, 30, 40, 50, 70]: Creates a Python list with elements 10, 30, 40, 50, and 70.
print(np.setdiff1d(array1, array2)): The np.setdiff1d function returns the sorted set difference between the two input arrays, which consists of elements that are in ‘array1’ but not in ‘array2’. In this case, the set difference is [0, 20, 60, 80], so the output will be [ 0 20 60 80].
For more Practice: Solve these Related Problems:
- Compute the set difference between two arrays using np.setdiff1d and verify that no elements from the second array appear.
- Create a custom function that performs set difference on unsorted arrays.
- Compare the output of np.setdiff1d with manual list comprehension filtering for validation.
- Apply set difference on arrays with duplicate values to ensure the result is distinct and sorted.
Go to:
PREV : Unique Elements of Array
NEXT : Set Exclusive-Or of Arrays
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.