NumPy: Get the values and indices of the elements that are bigger than 10 in a given array
NumPy: Array Object Exercise-31 with Solution
Elements >10 & Their Indices
Write a NumPy program to get the values and indices of the elements that are bigger than 10 in a given array.
Pictorial Presentation:
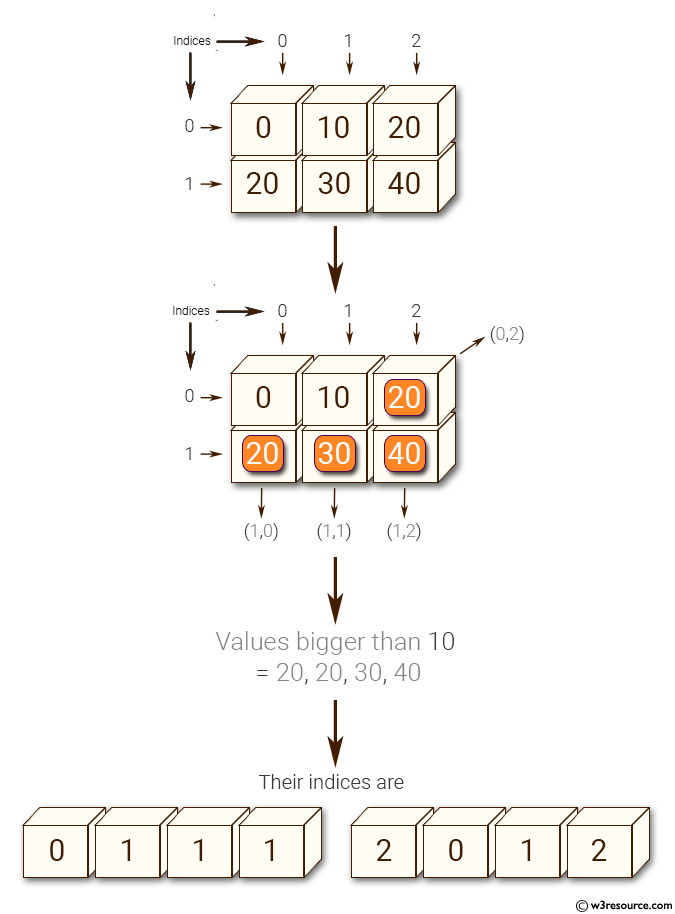
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a NumPy array
x = np.array([[0, 10, 20], [20, 30, 40]])
# Displaying the original array
print("Original array: ")
print(x)
# Filtering and displaying values in the array that are greater than 10
print("Values bigger than 10 =", x[x > 10])
# Displaying indices of elements that are greater than 10 using np.nonzero()
print("Their indices are ", np.nonzero(x > 10))
Sample Output:
Original array: [[ 0 10 20] [20 30 40]] Values bigger than 10 = [20 20 30 40] Their indices are (array([0, 1, 1, 1]), array([2, 0, 1, 2]))
Explanation:
In the above code –
x = np.array([[0, 10, 20], [20, 30, 40]]): x is a 2D NumPy array with two rows and three columns.
print("Values bigger than 10 =", x[x>10]): This line prints the extracted values that are greater than 10.
print("Their indices are ", np.nonzero(x > 10)): This line prints the indices of the values greater than 10 in the array x.
Python-Numpy Code Editor:
Previous: Write a NumPy program to sort pairs of first name and last name return their indices. (first by last name, then by first name).
Next: Write a NumPy program to save a NumPy array to a text file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/numpy/python-numpy-exercise-31.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics