NumPy: Create an array of 10's with the same shape and type of a given array
Array of 10s with Given Shape
Write a NumPy program to create an array of 10's with the same shape and type as the given array.
Sample array: x = np.arange(4, dtype=np.int64).
Pictorial Presentation:
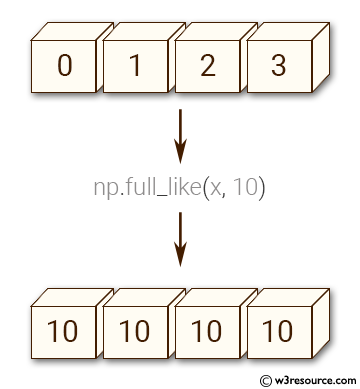
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a NumPy array with values ranging from 0 to 3 using arange method and specifying the data type as int64
x = np.arange(4, dtype=np.int64)
# Creating a new array 'y' with the same shape and type as array 'x' but filled with the value 10 using full_like method
y = np.full_like(x, 10)
# Printing the new array 'y'
print(y)
Sample Output:
[10 10 10 10]
Explanation:
x = np.arange(4, dtype=np.int64): This line creates a NumPy array ‘x’ containing elements from 0 to 3 (4-1) with a data type of np.int64 (64-bit integer).
y = np.full_like(x, 10): This line creates a new NumPy array ‘y’ with the same shape and data type as ‘x’, but filled with the value 10.
For more Practice: Solve these Related Problems:
- Create an array of 10s that matches the shape and dtype of a given array using broadcasting.
- Write a function that replicates the shape of an input array and fills it entirely with the number 10.
- Compare an array of 10s generated with np.ones multiplied by 10 to one created with np.full.
- Validate that the resulting array’s dimensions and type exactly mirror those of the reference array.
Go to:
PREV : 3x5 Array Filled with 2s
NEXT : 3D Array with Diagonal Ones
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.