NumPy: Move the specified axis backwards, until it lies in a given position
Move Axis to Desired Position
Write a NumPy program to move the specified axis backwards, until it lies in a given position.
Move the following 3rd array axes to first position.
(2,3,4,5)
Pictorial Presentation:
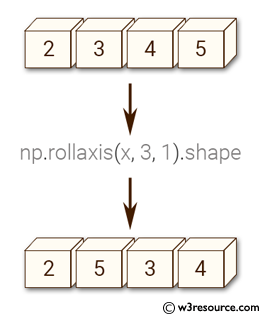
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a NumPy array filled with ones of shape (2, 3, 4, 5)
x = np.ones((2, 3, 4, 5))
# Using rollaxis to roll the axis at index 3 (last axis) to index 1
# Printing the shape of the array after rolling the axis from index 3 to index 1
print(np.rollaxis(x, 3, 1).shape)
Sample Output:
(2, 5, 3, 4)
Explanation:
In the above code -
x = np.ones((2, 3, 4, 5)): This part creates a 4D array x with the given shape (2, 3, 4, 5), filled with ones.
print(np.rollaxis(x, 3, 1).shape): The np.rollaxis() function takes three arguments: the input array, the axis to be rolled, and the axis to be rolled to. In this case, it rolls axis 3 (the fourth axis) to the position of axis 1 (the second axis). As a result, the shape of the new array is (2, 5, 3, 4).
For more Practice: Solve these Related Problems:
- Write a NumPy program to move a specified axis of a multi-dimensional array to the front using np.moveaxis.
- Shift the third axis of a 4D array to the first position and validate the resulting dimensions.
- Implement a function that repositions a chosen axis to a given target index within the array.
- Reorder the axes of an array so that the originally last axis becomes the second, using built-in functions.
Go to:
PREV : Move Array Axes to Alternate Positions
NEXT : Inputs as Arrays with 1+ Dimensions
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.