NumPy: Split an array of 14 elements into 3 arrays
Split 14 Elements into 3 Arrays
Write a NumPy program to split an array of 14 elements into 3 arrays, each with 2, 4, and 8 elements in the original order.
Sample array: [ 1 2 3 4 5 6 7 8 9 10 11 12 13 14]
Pictorial Presentation:
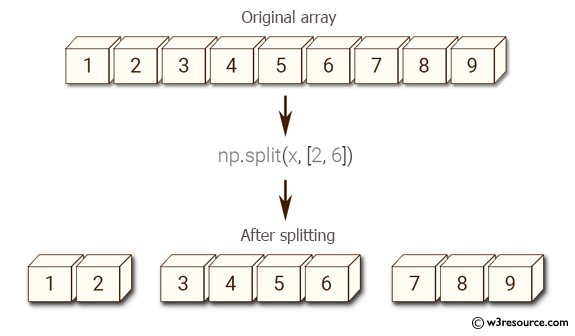
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a NumPy array 'x' containing numbers from 1 to 14 using np.arange
x = np.arange(1, 15)
# Displaying the original array 'x'
print("Original array:", x)
# Splitting the array 'x' into sub-arrays at specified indices using np.split
# The split points are at indices 2 and 6
# It creates three sub-arrays: [1, 2], [3, 4, 5, 6], and [7, 8, 9, 10, 11, 12, 13, 14]
print("After splitting:")
print(np.split(x, [2, 6]))
Sample Output:
Original array: [ 1 2 3 4 5 6 7 8 9 10 11 12 13 14] After splitting: [array([1, 2]), array([3, 4, 5, 6]), array([ 7, 8, 9, 10, 11, 12, 13, 14])]
Explanation:
x = np.arange(1, 15): This line creates a 1D array x containing the integers 1 to 14.
print(np.split(x, [2, 6])): The np.split() function is used to split the array x into multiple subarrays. The split indices are provided as a list [2, 6]. This means that the array x will be split into three subarrays: from the beginning to index 2 (exclusive), from index 2 to index 6 (exclusive), and from index 6 to the end.
For more Practice: Solve these Related Problems:
- Write a NumPy program to split an array of 14 elements into segments of sizes 2, 4, and 8 using np.split.
- Create a function that dynamically splits an array based on provided segment lengths and validates each segment.
- Test splitting an array using np.array_split and compare the result with manual slicing techniques.
- Handle edge cases by splitting an array into uneven segments and ensuring the order is maintained.
Go to:
PREV : Depth-Wise Conversion of 1D to 2D
NEXT : Split 4x4 Array Along Second Axis
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.