NumPy: Combine a one and a two dimensional array together and display their elements
Combine 1D and 2D Arrays
Write a NumPy program to combine a one and two dimensional array together and display their elements.
Pictorial Presentation:
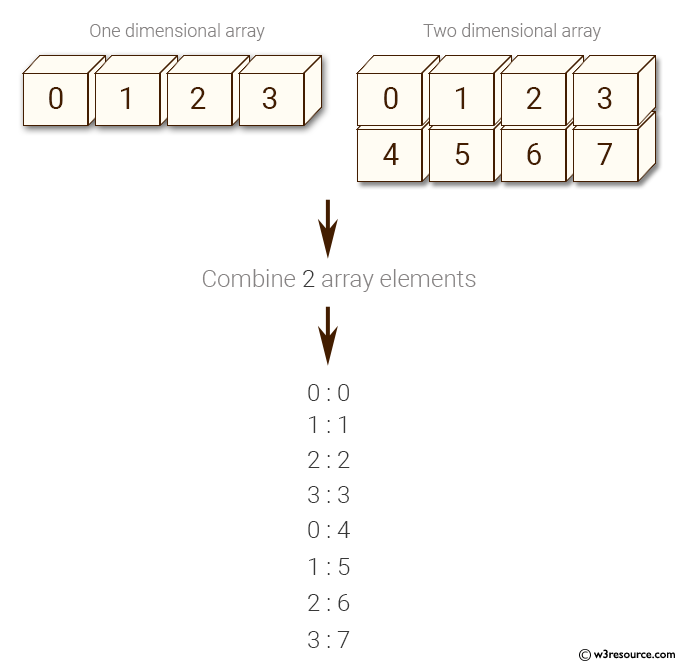
Sample Solution:
Python Code:
# Importing the NumPy library and aliasing it as 'np'
import numpy as np
# Creating a 1-dimensional array 'x' with values from 0 to 3
x = np.arange(4)
# Printing a message indicating the array 'x' is one-dimensional
print("One dimensional array:")
# Printing the 1-dimensional array 'x'
print(x)
# Creating a 2-dimensional array 'y' with values from 0 to 7 and reshaping it to a 2x4 array
y = np.arange(8).reshape(2, 4)
# Printing a message indicating the array 'y' is two-dimensional
print("Two dimensional array:")
# Printing the 2-dimensional array 'y'
print(y)
# Using a loop with np.nditer to simultaneously iterate through elements of 'x' and 'y'
# Printing each pair of corresponding elements from 'x' and 'y'
for a, b in np.nditer([x, y]):
print("%d:%d" % (a, b), end=' ') # Printing pairs of elements from 'x' and 'y' together
# Printing a newline character to separate the output
print()
Sample Output:
One dimensional array: [0 1 2 3] Two dimensional array: [[0 1 2 3] [4 5 6 7]] 0:0 1:1 2:2 3:3 0:4 1:5 2:6 3:7
Explanation:
In the above code –
np.arange(4): This function call creates a 1D NumPy array x with integers from 0 to 3.
np.arange(8).reshape(2,4): This line creates a 1D NumPy array with integers from 0 to 7 and then reshapes it into a 2x4 2D array y.
for a, b in np.nditer([x,y]):: This line initializes a loop using np.nditer to iterate over both arrays x and y simultaneously. Since x is a 1D array with shape (4,) and y is a 2D array with shape (2, 4), broadcasting rules make them compatible for iteration.
print("%d:%d" % (a,b),): Inside the loop, print() function prints each pair of corresponding elements from both arrays, separated by a colon.
For more Practice: Solve these Related Problems:
- Write a NumPy program to vertically stack a 1D array onto a 2D array using np.vstack and verify the new dimensions.
- Create a function that combines a 1D and a 2D array, then prints each element with its corresponding index.
- Concatenate a 1D array as an additional row or column to a 2D array and validate the resulting shape.
- Use np.hstack to merge a 1D array with a 2D array and compare the merged output with a manual approach.
Go to:
PREV : Multiply Array (3,4) Elements by 3
NEXT : Zeros Array with Multiple Column Types
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.